Build and Run
Description — Build and run a starter app to validate your install of Couchbase Lite on Android
Abstract — This content provides sample code and instructions that enable you to test your Couchbase Lite for android installation.
Introduction
The Getting Started app is a very basic Android app comprising a simple activity, which runs a set of Couchbase Lite CRUD functions, logging progress and output.
If you had problems, or just want more information on the app, there’s more below.
Those errors at the end of the run? They are because no Sync Gateway was running. That’s optional code — see below. Comment-it-out or remove it if you aren’t planning on running Sync Gateway.
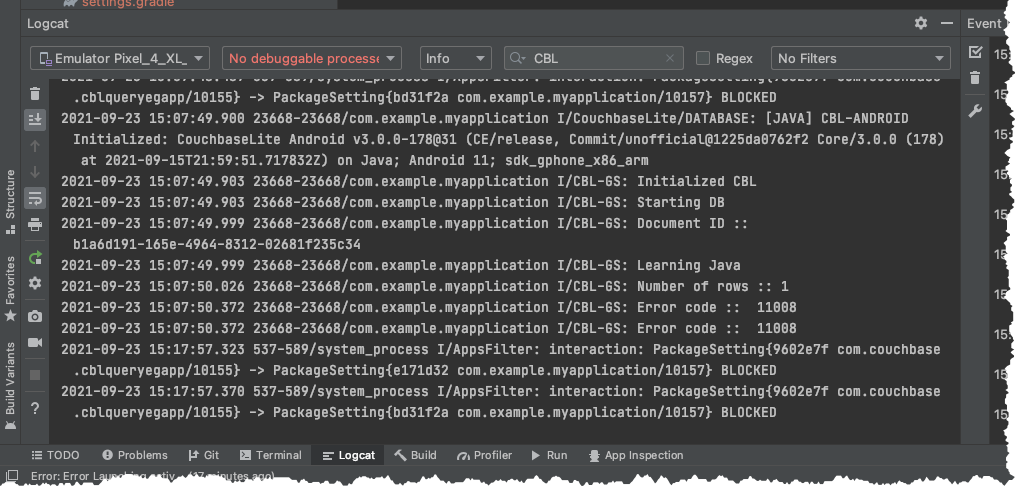
Getting Started App
The Getting Started app shows examples of the essential Couchbase for Android CRUD operations, including:
-
Create database
-
Create and save a document
-
Retrieve a document
-
Update a document
-
Query documents
-
Configure a replication
-
Initiate a replication
Whilst no exemplar of a real application, it will give you a good idea how to get started using Couchbase Lite.
Add the App to Your Own Project
-
Create a new 'empty activity' project in Android Studio
-
Open the
MainActivity
-
Delete the exiting generated content
-
Paste-in the code show in Example 1
-
Build and run.
You should see the document ID and property printed to the console. The document was successfully persisted to the database.
-
Kotlin
-
Java
class MainActivity : AppCompatActivity() {
private var TAG = "CBL-GS"
private var cntx: Context = this
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// One-off initialization
CouchbaseLite.init(cntx)
Log.i(TAG,"Initialized CBL")
//
// <.> Create a database
Log.i(TAG, "Starting DB")
val cfg = DatabaseConfigurationFactory.create()
val database = Database( "mydb", cfg)
(1)
// Create a new document (i.e. a record) in the database.
var mutableDoc = MutableDocument().setFloat("version", 2.0f).setString("type", "SDK")
(2)
// Save it to the database.
database.save(mutableDoc)
(3)
// Retrieve and update a document.
mutableDoc = database.getDocument(mutableDoc.id)!!.toMutable().setString("language", "Java")
database.save(mutableDoc)
(4)
// Retrieve immutable document and log the document ID
// generated by the database and some document properties
val document = database.getDocument(mutableDoc.id)!!
Log.i(TAG, "Document ID :: ${document.id}")
Log.i(TAG, "Learning ${document.getString("language")}")
(5)
// Create a query to fetch documents of type SDK.
val rs = QueryBuilder.select(SelectResult.all())
.from(DataSource.database(database))
.where(Expression.property("type").equalTo(Expression.string("SDK")))
.execute()
Log.i(TAG, "Number of rows :: ${rs.allResults().size}")
// (6)
// OPTIONAL -- if you have Sync Gateway Installed you can try replication too
// Create a replicator to push and pull changes to and from the cloud.
// Be sure to hold a reference somewhere to prevent the Replicator from being GCed
val replicator = Replicator(
ReplicatorConfigurationFactory.create(
database = database,
target = URLEndpoint(URI("ws://localhost:4984/getting-started-db")),
type = ReplicatorType.PUSH_AND_PULL,
authenticator = BasicAuthenticator("sync-gateway", "password".toCharArray())
)
)
// Listen to replicator change events.
// Version using Kotlin Flows to follow shortly ...
replicator.addChangeListener { change ->
val err = change.status.error
if (err != null) {
Log.i(TAG, "Error code :: ${err.code}")
}
}
// Start replication.
replicator.start()
jsonstuff(argDb = database)
}
fun jsonstuff (argDb: Database) {
var array1: MutableArray = MutableArray().setArray(1, ["a","b"])
var arrayJson1 = array1.to
}
}
public class MainActivity extends AppCompatActivity {
private String TAG = "CBL-GS";
private Context cntx = this;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// One-off initialization
CouchbaseLite.init(cntx);
Log.i(TAG,"Initialized CBL");
//
(1)
// Create a database
Log.i(TAG, "Starting DB");
DatabaseConfiguration cfg = new DatabaseConfiguration();
Database database = null;
try {
database = new Database( "mydb", cfg);
} catch (CouchbaseLiteException e) {
e.printStackTrace();
}
(2)
// Create a new document (i.e. a record) in the database.
MutableDocument mutableDoc =
new MutableDocument().setFloat("version", 2.0f)
.setString("type", "SDK");
(3)
// Save it to the database.
try {
database.save(mutableDoc);
} catch (CouchbaseLiteException e) {
e.printStackTrace();
}
(4)
// Retrieve and update a document.
mutableDoc =
database.getDocument(mutableDoc.getId())
.toMutable()
.setString("language", "Java");
try {
database.save(mutableDoc);
} catch (CouchbaseLiteException e) {
e.printStackTrace();
}
(5)
// Retrieve immutable document and log the document ID
// generated by the database and some document properties
Document document = database.getDocument(mutableDoc.getId());
Log.i(TAG, String.format("Document ID :: %s", document.getId()));
Log.i(TAG, String.format("Learning :: %s:", document.getString("language")));
(6)
// Create a query to fetch documents of type SDK.
try {
ResultSet rs =
QueryBuilder.select(SelectResult.all())
.from(DataSource.database(database))
.where(Expression.property("type").equalTo(Expression.string("SDK")))
.execute();
Log.i(TAG,
String.format("Number of rows :: %n",
rs.allResults().size()));
} catch (CouchbaseLiteException e) {
e.printStackTrace();
}
// (7)
// OPTIONAL -- if you have Sync Gateway Installed you can try replication too
// Create a replicator to push and pull changes to and from the cloud.
// Be sure to hold a reference somewhere to prevent the Replicator from being GCed
// BasicAuthenticator basAuth = new BasicAuthenticator("sync-gateway", "password".toCharArray());
//ReplicatorConfiguration replConfig =
// new ReplicatorConfiguration(database,
// URLEndpoint( URI("ws://localhost:4984/getting-started-db")),
// ReplicatorType.PUSH_AND_PULL,
// basAuth);
//
//Replicator replicator = new Replicator(replConfig);
// Listen to replicator change events.
// Version using Kotlin Flows to follow shortly ...
//replicator.addChangeListener(new ReplicatorChangeListener() {
// @Override
// public void changed(@NonNull ReplicatorChange change) {
// if (change.getStatus().getError() != null) {
// Log.i(TAG, "Error code :: ${err.code}");
// }
// }
// });
// Start replication.
// replicator.start();
}
}
1 | Create a database |
2 | Create a mutable document |
3 | Save document to database |
4 | Retrieve the document as mutable and change the language to Java and save it |
5 | Retrieve document as immutable and log it ID |
6 | Query the database output count and id to log |
7 | Optionally, initiate a replication |
Snags and Pitfalls
Mostly around Gradle and versions. You may find you need to change the project settings to use Java 11 for instance.
Using this app with Sync Gateway and Couchbase Server obviously requires you have, or install, working versions of both. See also — Install Sync Gateway
Minification
An application that enables minification must ensure that certain pieces of Couchbase Lite library code are not changed — see Example 2 for a near-minimal rule set that retains the needed code:
Kotlin-keep class com.couchbase.lite.ConnectionStatus { <init>(...); }
-keep class com.couchbase.lite.LiteCoreException { static <methods>; }
-keep class com.couchbase.lite.internal.core.C4* {
static <methods>;
<fields>;
<init>(...);
}