Data Sync Peer-to-Peer
Description — Couchbase Lite’s Peer-to-Peer Synchronization enables edge devices to synchronize securely without consuming centralized cloud-server resources
Abstract — An introduction to Couchbase Lite’s peer-to-peer sync.
Related Content — API Reference | Passive Peer | Active Peer
Introduction
Couchbase Lite’s Peer-to-Peer synchronization solution offers secure storage and bidirectional data synchronisation between edge devices without needing a centralized cloud-based control point.
Couchbase Lite’s Peer-to-Peer data synchronization provides:
-
Instant WebSocket-based listener for use in Peer-to-Peer applications communicating over IP-based networks
-
Simple application development, enabling sync with a short amount of code
-
Optimized network bandwidth usage and reduced data transfer costs with Delta Sync support
-
Securely sync data with built-in support for Transport Layer Security (TLS) encryption and authentication support
-
Document management. Reducing conflicts in concurrent writes with built-in conflict management support
-
Built-in network resiliency
Overview
Peer-to-Peer synchronization requires one Peer to act as the Listener to the other Peer’s replicator.
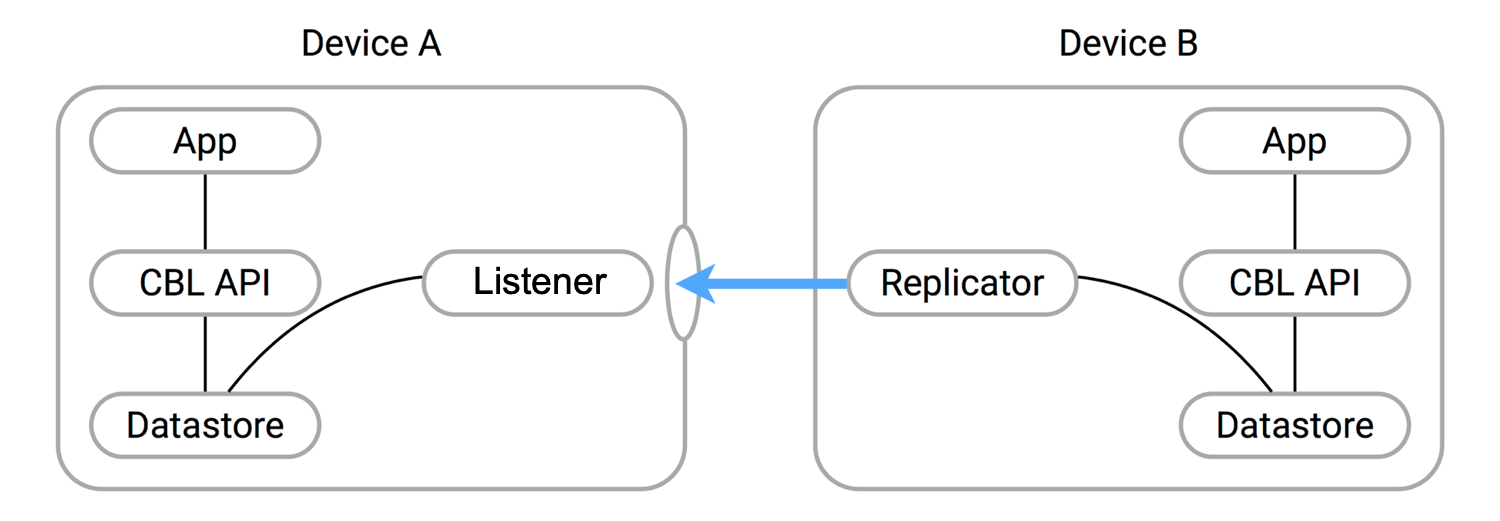
Peer-to-Peer synchronization requires one Peer to act as the Listener to the other Peer’s replicator. Therefore, to use Peer-to-Peer synchronization in your application, you must configure one Peer to act as a Listener using the Couchbase Listener API, the most important of which include URLEndpointListener and URLEndpointListenerConfiguration.
-
Configure the Listener (passive peer, or server)
-
Initialize the Listener, which listens for incoming WebSocket connections (on a user-defined, or auto-selected, port)
-
Configure a replicator (active peer, or client)
-
Use some form of discovery phase, perhaps with a zero-config protocol , or use known URL endpoints, to identify a Listener
-
Point the replicator at the Listener
-
Initialize the replicator
-
Replicator and Listener engage in the configured security protocol exchanges to confirm connection
-
If connection is confirmed then replication will commence, synchronizing the two data stores.
Here you can see configuration involves a Passive Peer and an Active Peer and a user-friendly Listener configuration in Basic Setup
You can also learn how to implement Peer-to-Peer synchronization by referring to our tutorial — see: Getting Started with Peer-to-Peer Synchronization.
Features
Couchbase Lite for C#.Net’s Peer-to-Peer synchronization solution provides support for cross-platform synchronization, for example, between Android and iOS devices.
Each listener instance serves one Couchbase Lite database. However, there is no hard limit on the number of Listener instances you can associate with a database.
Having a Listener on a database still allows you to open replications to the other clients. For example, a Listener can actively begin replicating to other Listeners while listening for connections. These replications can be for the same or a different database.
The Listener will automatically select a port to use or a user-specified port. It will also listen on all available networks, unless you specify a specific network.
Security
Couchbases Lite’s Peer-to-Peer synchronization supports encryption and authentication over TLS with multiple modes, including:
-
No encryption, for example, clear text.
-
CA Cert
-
Self-signed Cert
-
Anonymous Self-signed — an auto-generated anonymous TLS identity is generated if no identity is specified. This TLS identity provides encryption but not authentication.
Any self-signed certificates generated by the convenience API are stored in secure storage.
The replicator (client) can handle certificates pinned by the Listener for authentication purposes.
Support is also provided for basic authentication using username and password credentials. Whilst this can be used in clear text mode, developers are strongly advised to use TLS encryption.
For testing and development purposes, support is provided for the client (active, replicator) to skip verification of self-signed certificates; this mode should not be used in production.
Error Handling
When a Listener is stopped, then all connected replicators are notified by a WebSocket error. Your application should distinguish between transient and permanent connectivity errors.
Delta Sync
Optional delta-sync support is provided but is inactive by default.
Delta-sync can be enabled on a per-replication basis provided that the databases involved are also configured to permit it. Statistics on delta-sync usage are available, including the total number of revisions sent as deltas.
Basic Setup
You can configure a Peer-to-Peer synchronization with just a short amount of code as shown here in Example 2 and Example 3.
This simple listener configuration will give you a listener ready to participate in an encrypted synchronization with a replicator providing a valid user name and password.
var endpointConfig = new URLEndpointListenerConfiguration(new[] { collection }); (1)
endpointConfig.Authenticator =
new ListenerPasswordAuthenticator(
(sender, username, password) =>
{
// ValidatePassword can make use of the SecureString class
// to the desired level of security (or just convert it to string
// if no intense security is required)
return username == "valid.user" && ValidatePassword(password);
}
); (2)
var listener = new URLEndpointListener(endpointConfig); (3)
listener.Start(); (4)
1 | Initialize the Listener configuration |
2 | Configure the client authenticator to require basic authentication |
3 | Initialize the Listener |
4 | Start the Listener |
This simple replicator configuration will give you an encrypted, bi-directional Peer-to-Peer synchronization with automatic conflict resolution.
var endpointConfig = new URLEndpoint(new Uri("wss://listener.com:4984/otherDB")); (1)
var replConfig = new ReplicatorConfiguration(endpointConfig); (2)
replConfig.AddCollection(collection);
replConfig.AcceptOnlySelfSignedServerCertificate = true; (3)
replConfig.Authenticator =
new BasicAuthenticator("valid.user", "valid.password.string"); (4)
var replicator = new Replicator(replConfig); (5)
replicator.Start(); (6)
1 | Get the Listener’s endpoint. Here we use a known URL, but it could be a URL established dynamically in a discovery phase. |
2 | Initialize the replicator configuration with the database to be synchronized and the Listener it is to synchronize with |
3 | Configure the replicator to expect a self-signed certificate from the Listener |
4 | Configure the replicator to present basic authentication credentials if the Listener prompts for them (client authentication is optional) |
5 | Initialize the replicator |
6 | Start the replicator |
API Highlights
URLEndpointListener
The URLEndpointListener
is the listener for peer-to-peer synchronization.
It acts like a passive replicator, in the same way that Sync Gateway does in a 'standard' replication.
On the client side, the listener’s endpoint is used to point the replicator to the listener.
Core functionalities of the listener are:
-
Users can initialize the class using a URLEndpointListenerConfiguration object.
-
The listener can be started, or can be stopped.
-
Once the listener is started, a total number of connections or active connections can be checked.
API Reference: URLEndpointListener
URLEndpointListenerConfiguration
Use this to create a configuration object you can then use to initialize the listener.
- Port
-
This is the port that the listener will listen to.
If the port is null or zero, the listener will auto-assign an available port to listen on.
Default value is null or zero depending on platform. When the listener is not started, the port is null (or zero if the platform requires).
- Network Interface
-
Use this to select a specific Network Interface to use, in the form of the IP Address or network interface name.
If the network interface is specified, only that interface wil be used.
If the network interface is not specified, all available network interfaces will be used.
The value is null if the listener is not started.
- disableTLS
-
You can use URLEndpointListenerConfiguration's DisableTLS method to disable TLS communication if necessary
The
disableTLS
setting must be 'false' when Client Cert Authentication is required.Basic Authentication can be used with, or without, TLS.
DisableTLS works in conjunction with
TLSIdentity
, to enable developers to define the key and certificate to be used.-
If
disableTLS
is true — TLS communication is disabled and TLS identity is ignored. Active peers will use thews://
URL scheme used to connect to the listener. -
If
disableTLS
is false or not specified — TLS communication is enabled.Active peers will use the
wss://
URL scheme to connect to the listener.
API Reference: DisableTLS
-
- tlsIdentity
-
Use URLEndpointListenerConfiguration's TlsIdentity method to configure the TLS Identity used in TLS communication.
If
TLSIdentity
is not set, then the listener uses an auto-generated anonymous self-signed identity (unlessdisableTLS = true
). Whilst the client cannot use this to authenticate the server, it will use it to encrypt communication, giving a more secure option than non-TLS communication.The auto-generated anonymous self-signed identity is saved in secure storage for future use to obviate the need to re-generate it.
When the listener is not started, the identity is null. When TLS is disabled, the identity is always null.
API Reference: TlsIdentity
- authenticator
-
Use this to specify the authenticator the listener uses to authenticate the client’s connection request. This should be set to one of the following:
-
ListenerPasswordAuthenticator
-
ListenerCertificateAuthenticator
-
Null — there is no authentication.
API Reference: Authenticator
-
- readOnly
-
Use this to allow only pull replication. Default value is false.
- enableDeltaSync
-
The option to enable Delta Sync and replicate only changed data also depends on the delta sync settings at database level. The default value is false.
API Reference: URLEndpointListenerConfiguration
Security
Authentication
Peer-to-Peer sync supports Basic Authentication and TLS Authentication. For anything other than test deployments, we strongly encourage the use of TLS. In fact, Peer-to-Peer sync using URLEndpointListener is encrypted using TLS by default.
The authentication mechanism is defined at the endpoint level, meaning that it is independent of the database being replicated. For example, you may use basic authentication on one instance and TLS authentication on another when replicating multiple database instances.
The Minimum supported version of TLS is TLS 1.2. |
Peer-to-Peer synchronization using URLEndpointListener supports certificate based authentication of the server and-or Listener:
-
Replicator certificates can be: self signed, from trusted CA or anonymous (system generated).
-
Listeners certificates may be: self signed or trusted CA signed.
Where a TLS certificate is not explicitly specified for the Listener, the Listener implementation will generate anonymous certificate to use for encryption
-
The URLEndpointListener supports the ability to opt out of TLS encryption communication.
Active clients replicating with a URLEndpointListener have the option to skip validation of server certificates when the Listener is configured with self-signed certificates.
This option is ignored when dealing with CA certificates.
Using Secure Storage
TLS and its associated keys and certificates might require using secure storage to minimize the chances of a security breach. The implementation of this storage differs from platform to platform. Table 1 summarizes the secure storage used to store keys and certificates for C#.Net.
Key |
Value |
Platform |
Android |
Key Storage |
Android System KeyStore |
Certificate Storage |
Android System KeyStore |
Notes |
|
Reference |
|
Platform |
MacOS/iOS |
Key Storage |
KeyChain |
Certificate Storage |
KeyChain |
Notes |
Use kSecAttrLabel of the SecCertificate to store the TLSIdentity’s label |
Reference |
https://developer.apple.com/documentation/security/keychain_services |
Platform |
Java |
Key Storage |
User Specified KeyStore |
Certificate Storage |
User Specified KeyStore |
Notes |
|
Reference |
https://docs.oracle.com/javase/7/docs/api/java/security/KeyStore.html |
Platform |
.Net (excluding Xamarin) |
Key Storage |
Opaque; Keys are stored automatically by the runtime when storing the certificate with the PersistKeySet flag set. |
Certificate Storage |
User specified X509Store |
Notes |
|
Reference |
Platform |
Xamarin |
Key Storage |
RSACryptoServiceProvider provided by Xamarin. |
Certificate Storage |
User specified X509Store |
Notes |
|
Reference |