Nested Operators and Expressions
- reference
In SQL++, nested operators and paths indicate expressions to access nested sub-documents within a JSON document or expression.
A nested expression may contain field selection operators, element selection operators, and array slicing operators.
nested-expr ::= field-expr | element-expr | slice-expr
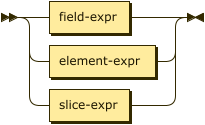
These special operators are needed to access the data because Couchbase documents can have nested objects and embedded arrays. A field selection operator is used to refer to a field in an object, and an element selection operator is used to refer to an element in an array. You can use a combination of these operators to access nested data at any depth in a document.
Field Selection
Field selection operators use the dot notation .
to refer to a child field, that is, a field one level down in a nested object.
Arguments
- expr
-
An expression resolving to an object.
- identifier
- escaped-identifier
-
An identifier which specifies the name of a field in the object.
- name-expr
-
An expression resolving to a string which specifies the name of a field in the object.
By default, field names are case sensitive.
To access a field case-insensitively, include the trailing i
.
Element Selection
Element selection operators use the bracket notation [ ]
to access an element inside a nested array.
Array Slicing
The array slicing operator enables you to get subsets or segments of an array.
Arguments
- expr
-
An expression resolving to an array.
- start-expr
-
A numeric expression specifying a start position in the array, counting from 0. Negative positions are counted backwards from the end of the array.
- end-expr
-
[Optional] A numeric expression specifying an end position in the array, counting from 0. Negative positions are counted backwards from the end of the array.
Return Value
A new subset of the source array, containing the elements from the start position to the end position minus 1. The element at the start position is included, while the element at the end position is not.
If the end position is omitted, all elements from the start position to the end of the source array are included.