Creating the Courses Collection
Your first application created a single student record for the student collection. In this part, you’re going to populate the course collection.
Populating the course details collection
You can use the same technique to build a store for the courses. Here’s a quick reminder of the course document structure:
-
art history
-
graphic design
-
fine art
{
"course-name": "art history",
"faculty": "fine art",
"credit-points" : 100
}
{
"course-name": "graphic design",
"faculty": "media and communication",
"credit-points" : 200
}
{
"course-name": "fine art",
"faculty": "fine art",
"credit-points" : 50
}
The code should be familiar to you; there’s not much difference between writing to the course collection and writing to the student collection; you just have more records to deal with:
import com.couchbase.client.java.Bucket;
import com.couchbase.client.java.Cluster;
import com.couchbase.client.java.Collection;
import com.couchbase.client.java.Scope;
import com.couchbase.client.java.json.JsonObject;
import java.time.Duration;
public class InsertCourses {
public static void main(String[] args) {
Cluster cluster = Cluster.connect("localhost",
"Administrator", "password");
Bucket bucket = cluster.bucket("student-bucket");
bucket.waitUntilReady(Duration.ofSeconds(10));
Scope scope = bucket.scope("art-school-scope");
Collection course_records = scope.collection("course-record-collection"); (1)
addCourse(course_records, "ART-HISTORY-000001", "art history", "fine art", 100);
addCourse(course_records, "FINE-ART-000002", "fine art", "fine art", 50);
addCourse(course_records, "GRAPHIC-DESIGN-000003", "graphic design", "media and communication", 200);
cluster.disconnect();
}
private static void addCourse(Collection collection, String id, String name,
String faculty, int creditPoints) {
JsonObject course = JsonObject.create()
.put("course-name", name)
.put("faculty", faculty)
.put("credit-points", creditPoints);
collection.upsert(id, course);
}
}
1 | Note that you’re now writing to a different collection. |
Make sure that you’ve created the course-collection in the admin console before you attempt to run the program.
|
You can use maven to run the application:
mvn exec:java -Dexec.mainClass="InsertCourses" -Dexec.cleanupDaemonThreads=false
Use the admin console to make sure the documents have been created in the correct collection.
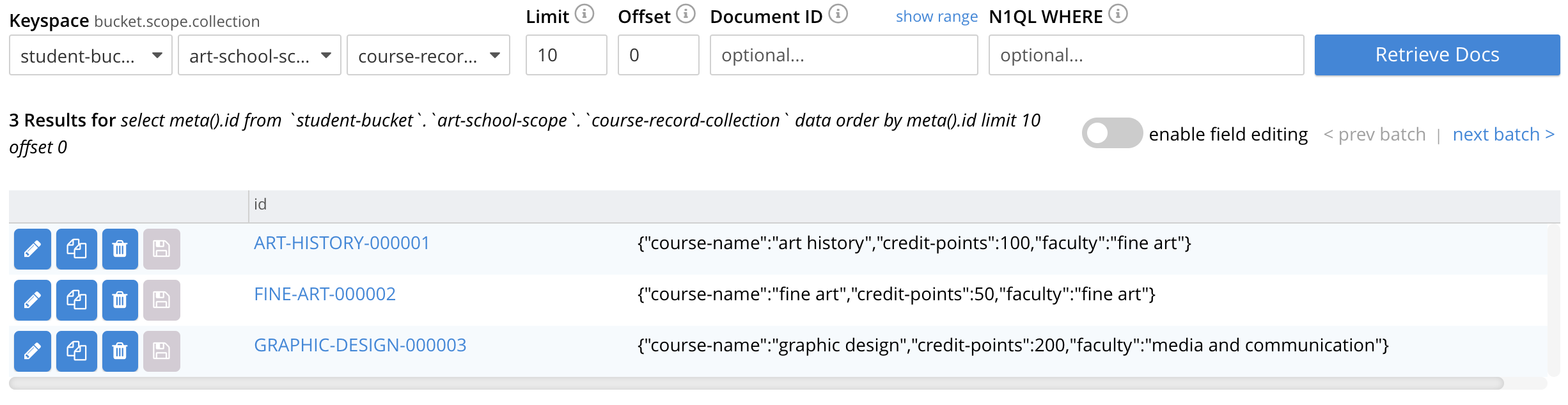
Next steps
So you’ve created a cluster, a bucket, a scope and two collections. You’ve also populated your collections with documents. Well, a database isn’t much use until we can retrieve information from it, which is what you’re going to take a look at in the next part.