Integrate a Custom Built Listener
Description — Couchbase Lite database peer-to-peer sync- integrate a custom built listener
Related Content — Peer-to-Peer
Overview
Enterprise Edition only
Peer-to-Peer Synchronization is an Enterprise Edition feature.
You must purchase the Enterprise License, which includes official Couchbase Support.
To use it in production (also see the FAQ).
|
This content covers how to integrate a custom MessageEndpointListener solution with Couchbase Lite to handle the data transfer, which is the sending and receiving of data. Where applicable, we discuss how to integrate Couchbase Lite into the workflow.
The following sections describe a typical Peer-to-Peer workflow.
Peer Discovery
Peer discovery is the first step. The communication framework will generally include a Peer discovery API for devices to advertise themselves on the network and to browse for other Peers.
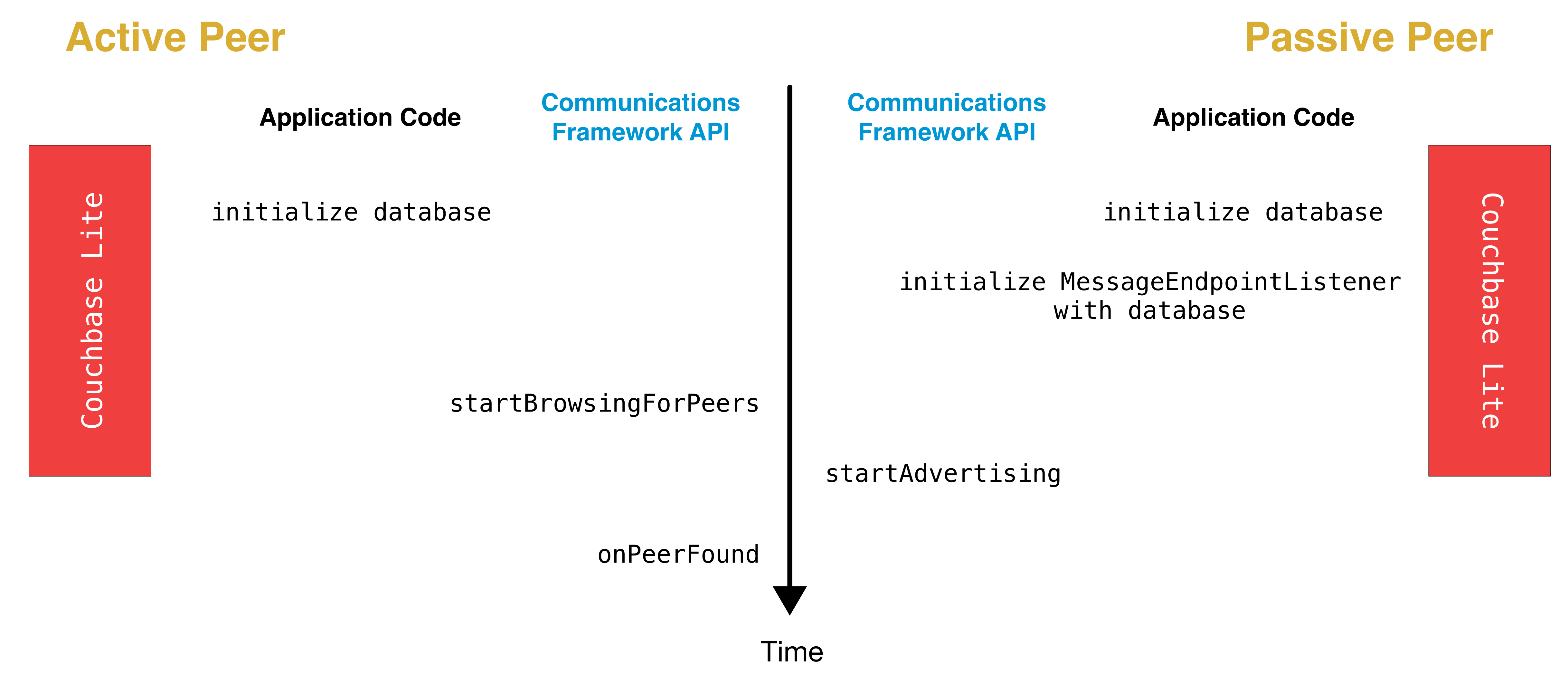
Passive Peer
In addition to initializing the database, the Passive Peer must initialize the MessageEndpointListener
.
The MessageEndpointListener
acts as a Listener for incoming connections.
var database = new Database("mydb");
var endpointConfig = new MessageEndpointListenerConfiguration(new[] { database.GetDefaultCollection() }, ProtocolType.MessageStream);
_messageEndpointListener = new MessageEndpointListener(endpointConfig);
Peer Selection and Connection Setup
Once a Peer device is found, the application code must decide whether it should establish a connection with that Peer. This step includes inviting a Peer to a session and Peer authentication.
This is handled by the Communication Framework.
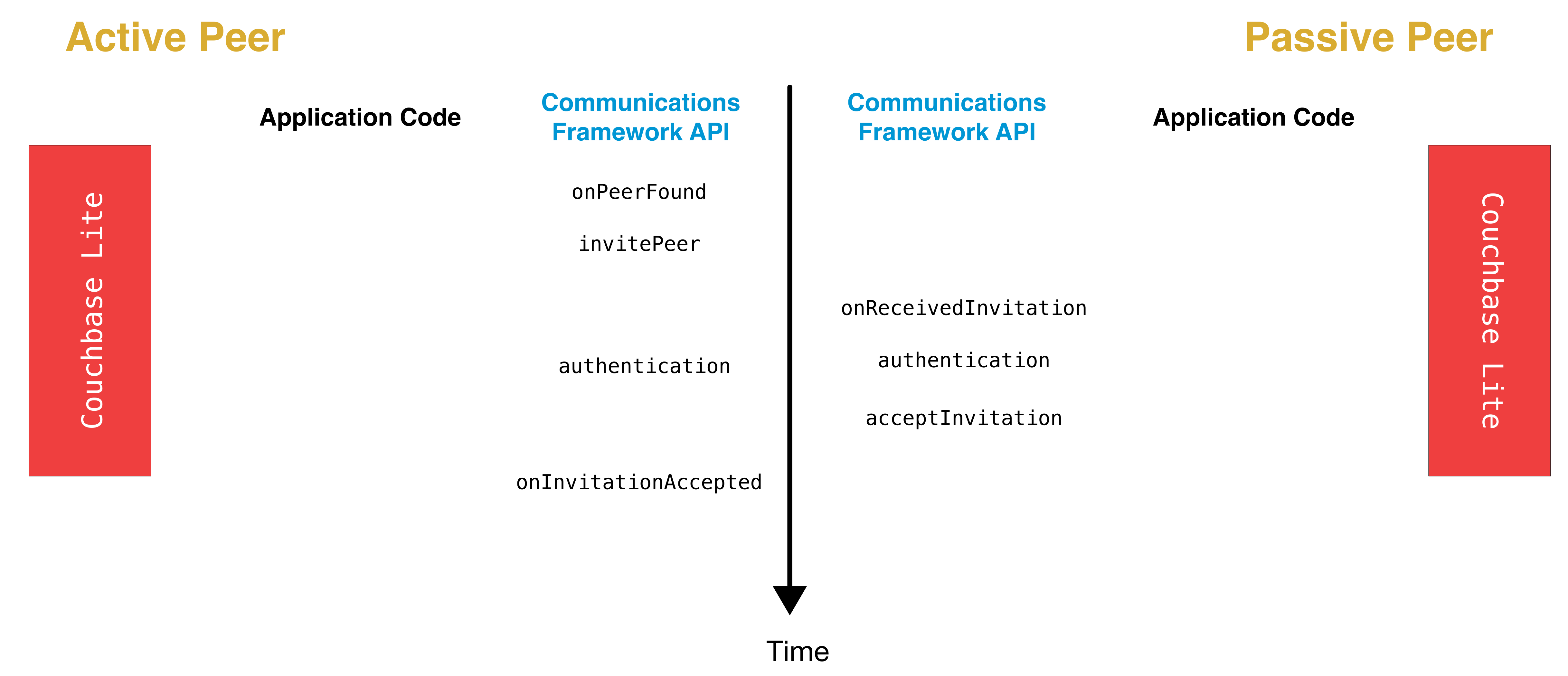
Once the remote Peer has been authenticated, the next step is to connect with that Peer and initialize the Message Endpoint API.
Replication Setup
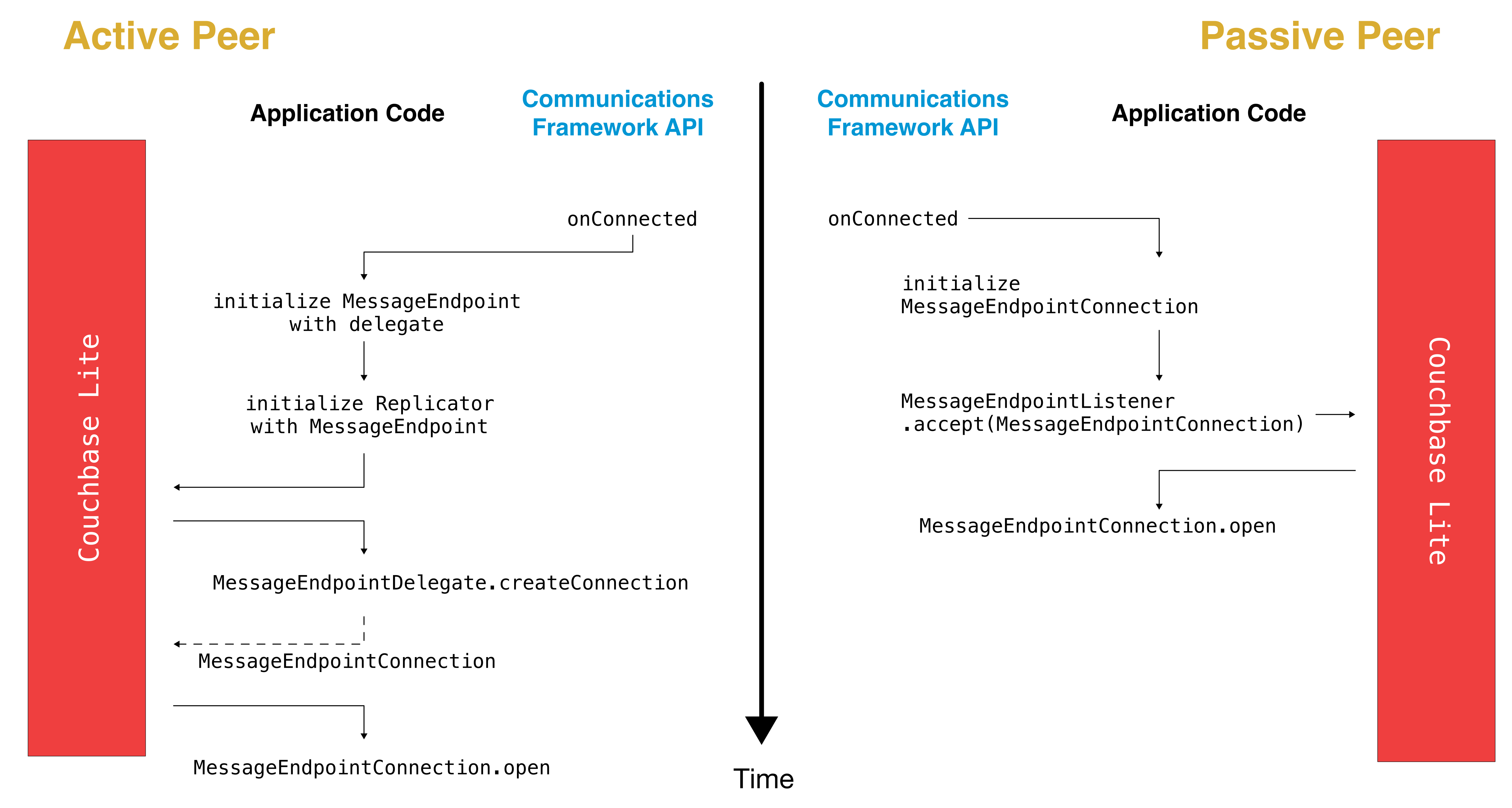
Active Peer
When the connection is established, the active Peer must instantiate a MessageEndpoint
object corresponding to the remote Peer.
var database = new Database("dbname");
// The delegate must implement the `IMessageEndpointDelegate` protocol.
var messageEndpointTarget = new MessageEndpoint(uid: "UID:123", target: "",
protocolType: ProtocolType.MessageStream, delegateObject: this);
The MessageEndpoint
initializer takes the following arguments.
-
uid
: a unique ID that represents the remote active Peer. -
target
: This represents the remote passive Peer and could be any suitable representation of the remote Peer. It could be an Id, URL etc. If using the MultiPeerConnectivity Framework, this could be the MCPeerID. -
protocolType
: specifies the kind of transport you intend to implement. There are two options.-
The default (
MessageStream
) means that you want to "send a series of messages", or in other words the Communication Framework will control the formatting of messages so that there are clear boundaries between messages. -
The alternative (
ByteStream
) means that you just want to send raw bytes over the stream and Couchbase should format for you to ensure that messages get delivered in full.Typically, the Communication Framework will handle message assembly and disassembly so you would use the
MessageType
option in most cases.
-
-
delegate
: the delegate that will implement theMessageEndpointDelegate
protocol, which is a factory forMessageEndpointConnection
.
Then, a Replicator
is instantiated with the initialized MessageEndpoint
as the target.
var replConfig = new ReplicatorConfiguration(messageEndpointTarget);
replConfig.AddCollection(database.GetDefaultCollection());
// Create the replicator object
var replicator = new Replicator(replConfig);
// Start the replicator
replicator.Start();
Next, Couchbase Lite will call back the application code through the MessageEndpointDelegate.createConnection
interface method.
When the application receives the callback, it must create an instance of MessageEndpointConnection
and return it.
/* implementation of MessageEndpointDelegate */
public IMessageEndpointConnection CreateConnection(MessageEndpoint endpoint)
{
var connection = new ActivePeerConnection(); /* implements MessageEndpointConnection */
return connection;
}
Next, Couchbase Lite will call back the application code through the MessageEndpointConnection.open
method.
/* implementation of MessageEndpointConnection */
public async Task Open(IReplicatorConnection connection)
{
_replicatorConnection = connection;
// await socket.Open(), etc
// throw MessagingException if something goes wrong
}
The connection argument is then set on an instance variable.
The application code must keep track of every ReplicatorConnection
associated with every MessageEndpointConnection
.
The MessageError
argument in the completion block specifies whether the error is recoverable or not.
If it is a recoverable error, the replicator will begin a retry process, creating a new MessageEndpointConnection
instance.
Passive Peer
After connection establishment on the Passive Peer, the first step is to initialize a new MessageEndpointConnection
and pass it to the listener.
This message tells the listener to accept incoming data from that Peer.
var connection = new PassivePeerConnection(); /* implements MessageEndpointConnection */
_messageEndpointListener?.Accept(connection);
messageEndpointListener
is the instance of the MessageEndpointListener
that was created in the first step (Peer Discovery)
Couchbase Lite will call the application code back through the MessageEndpointConnection.open
method.
/* implementation of MessageEndpointConnection */
public Task Open(IReplicatorConnection connection)
{
_replicatorConnection = connection;
// socket should already be open on the passive side
return Task.FromResult(true);
}
The connection
argument is then set on an instance variable.
The application code must keep track of every ReplicatorConnection
associated with every MessageEndpointConnection
.
At this point, the connection is established, and both Peers are ready to exchange data.
Push/Pull Replication
Typically, an application needs to send data and receive data. The directionality of the replication could be any of the following.
-
Push only: The data is pushed from the local database to the remote database.
-
Pull only: The data is pulled from the remote database to the local database.
-
Push and Pull: The data is exchanged both ways.
Usually, the remote is a Sync Gateway database identified through a URL. In Peer-to-Peer syncing, the remote is another Couchbase Lite database.
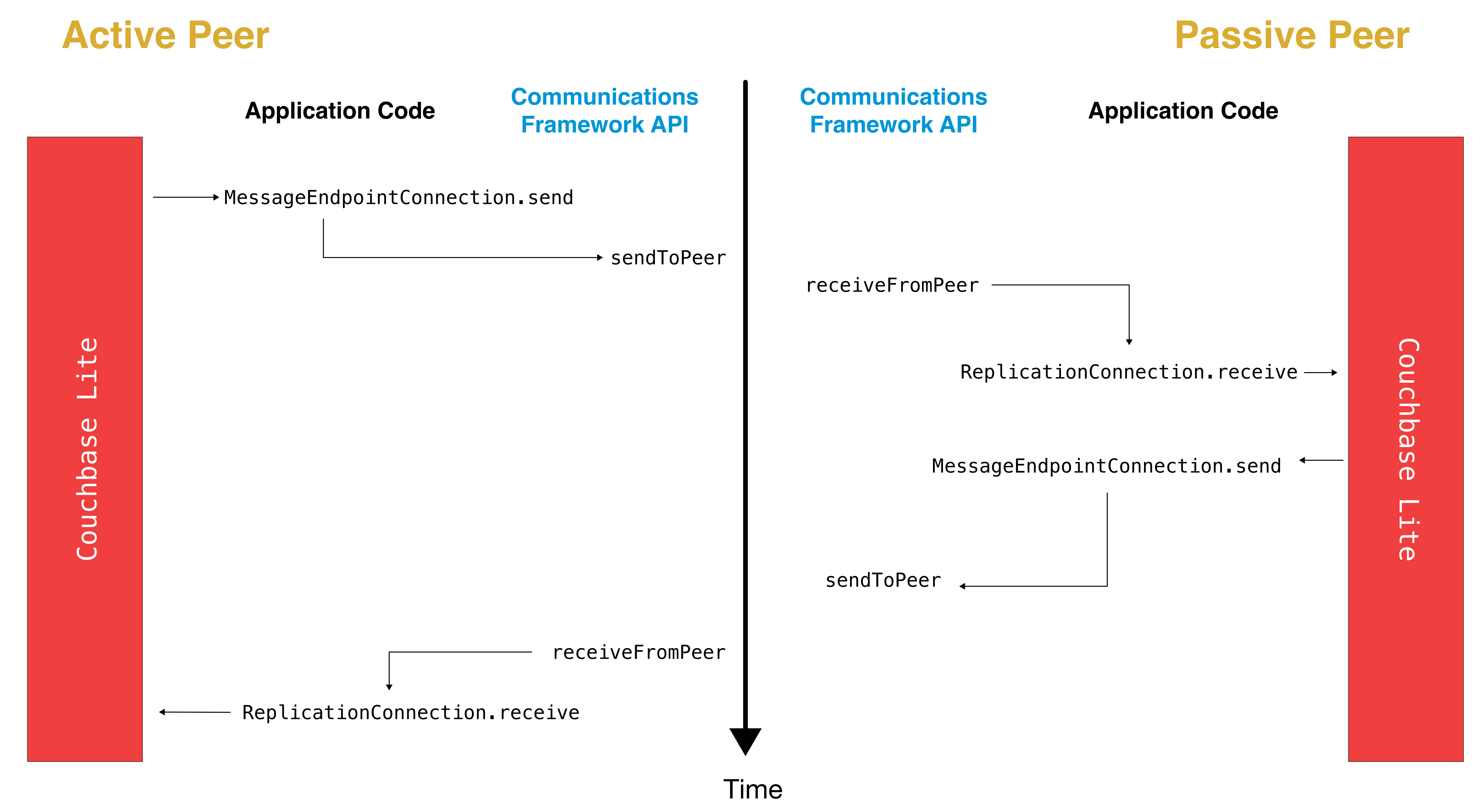
The replication lifecycle is handled through the MessageEndpointConnection
.
Active Peer
When Couchbase Lite calls back the application code through the MessageEndpointConnection.send
method, you should send that data to the other Peer using the communication framework.
/* implementation of MessageEndpointConnection */
public async Task Send(Message message)
{
var data = message.ToByteArray();
// await Socket.Send(), etc
// throw MessagingException if something goes wrong
}
Once the data is sent, call the completion block to acknowledge the completion.
You can use the MessageError
in the completion block to specify whether the error is recoverable.
If it is a recoverable error, the replicator will begin a retry process, creating a new MessageEndpointConnection
.
When data is received from the passive Peer via the Communication Framework, you call the ReplicatorConnection.receive
method.
var message = Message.FromBytes(data);
_replicatorConnection?.Receive(message);
The replication connection’s receive
method is called. Which then processes the data to persist to the local database.
Passive Peer
As in the case of the active Peer, the passive Peer must implement the MessageEndpointConnection.send
method to send data to the other Peer.
/* implementation of MessageEndpointConnection */
public async Task Send(Message message)
{
var data = message.ToByteArray();
// await Socket.Send(), etc
// throw MessagingException if something goes wrong
}
Once the data is sent, call the completion block to acknowledge the completion.
You can use the MessageError
in the completion block to specify whether the error is recoverable.
If it is a recoverable error, the replicator will begin a retry process, creating a new MessageEndpointConnection
.
When data is received from the active Peer via the Communication Framework, you call the ReplicatorConnection.receive
method.
var message = Message.FromBytes(data);
_replicatorConnection?.Receive(message);
Connection Teardown
When a Peer disconnects from a Peer-to-Peer network, all connected Peers are notified. The disconnect notification is a good opportunity to close and remove a replication connection. The steps to Teardown the connection are slightly different depending on whether the active or passive Peer disconnects first. We will cover each case below.
Initiated by Active Peer
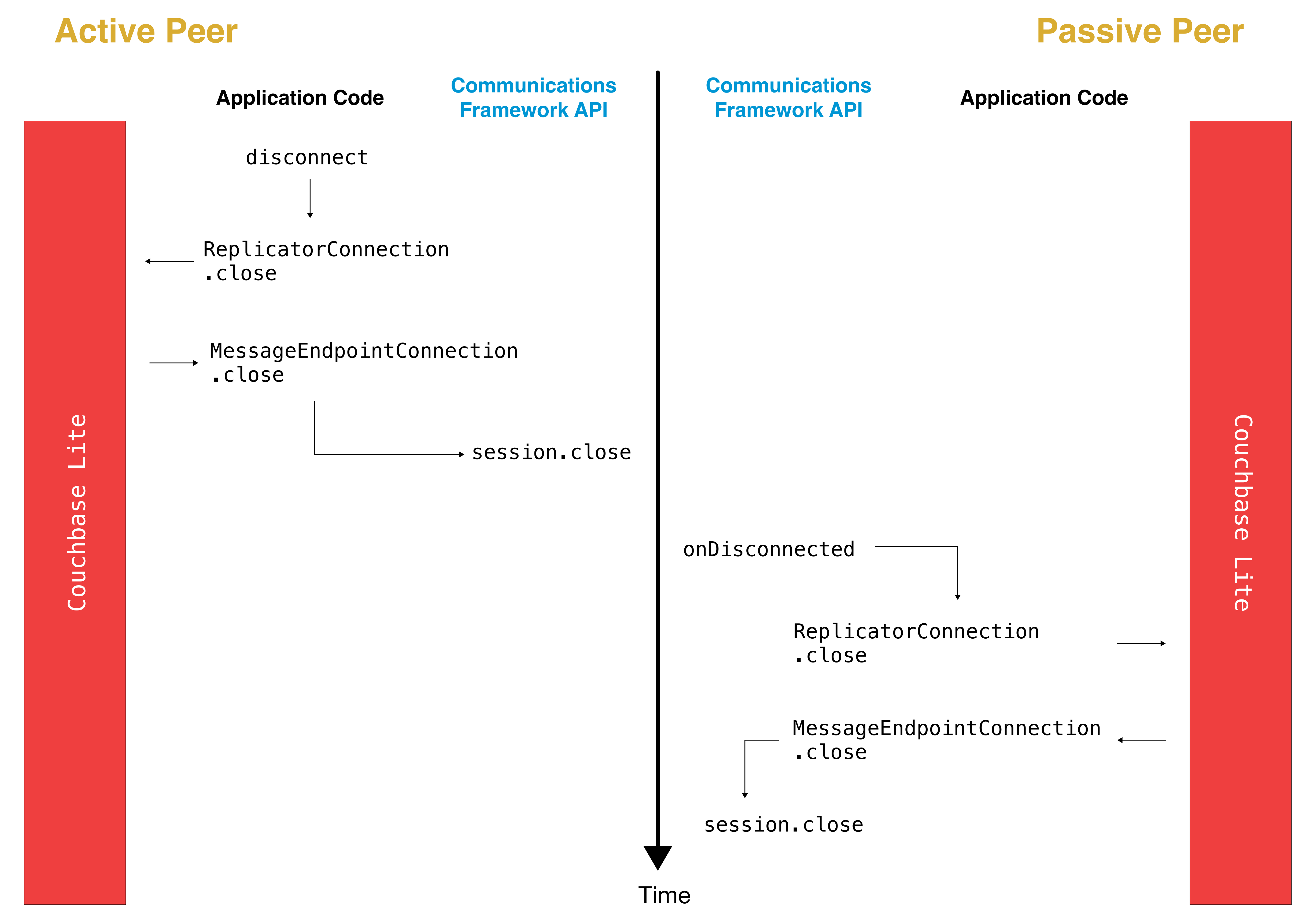
Active Peer
When an active Peer disconnects, it must call the ReplicatorConnection.close
method.
_replicatorConnection?.Close(null);
Then, Couchbase Lite will call back your code through the MessageEndpointConnection.close
to allow the application to disconnect with the Communication Framework.
/* implementation of MessageEndpointConnection */
public async Task Close(Exception error)
{
// await socket.Close, etc (or do nothing if already closed)
// throw MessagingException if something goes wrong (though
// since it is "close" nothing special will happen)
}
Passive Peer
When the passive Peer receives the corresponding disconnect notification from the Communication Framework, it must call the ReplicatorConnection.close
method.
_replicatorConnection?.Close(null);
Then, Couchbase Lite will call back your code through the MessageEndpointConnection.close
to allow the application to disconnect with the Communication Framework.
/* implementation of MessageEndpointConnection */
public async Task Close(Exception error)
{
// await socket.Close, etc (or do nothing if already closed)
// throw MessagingException if something goes wrong (though
// since it is "close" nothing special will happen)
}
Initiated by Passive Peer
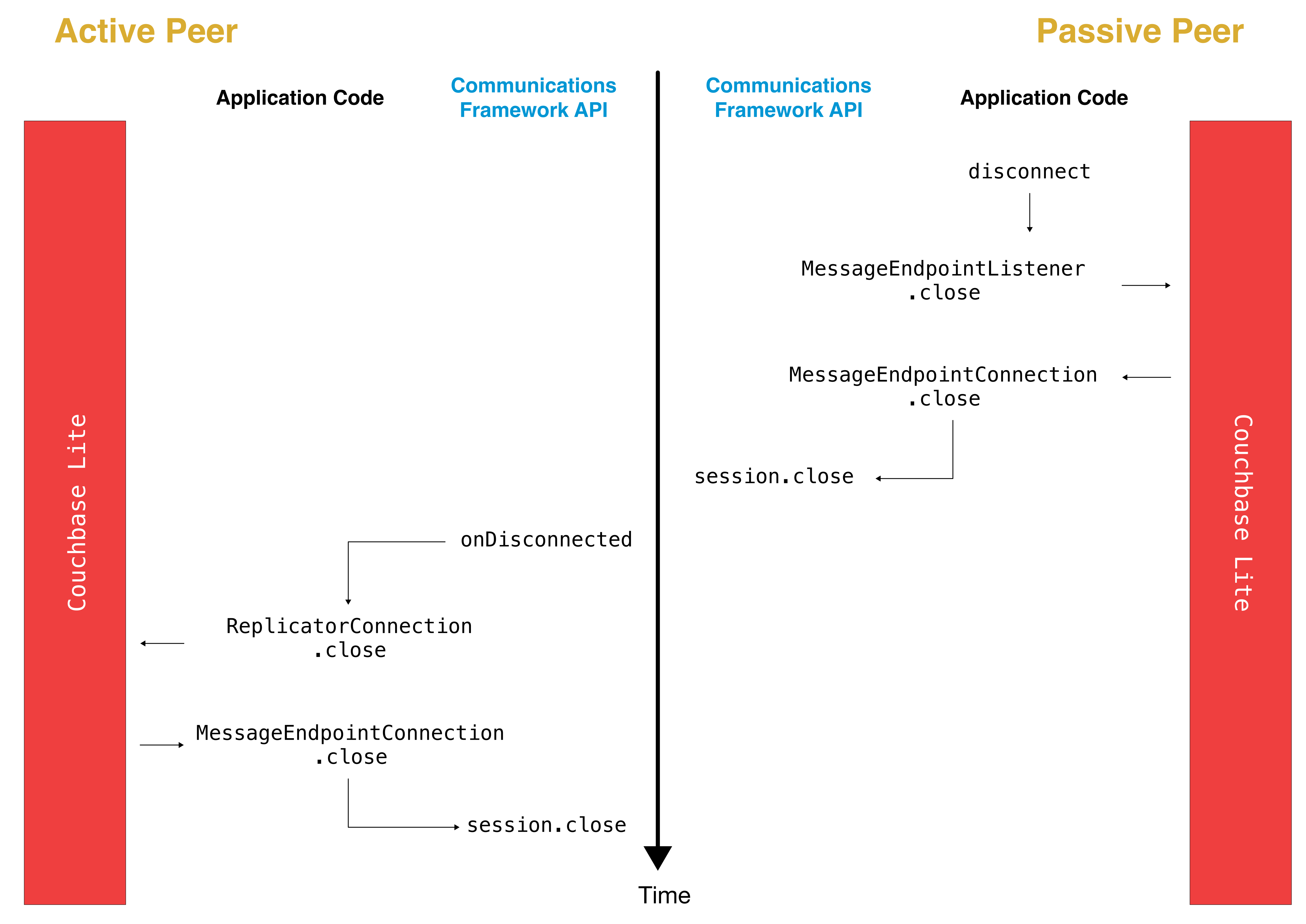
Passive Peer
When the passive disconnects, it must class the MessageEndpointListener.closeAll
method.
_messageEndpointListener?.CloseAll();
Then, Couchbase Lite will call back your code through the MessageEndpointConnection.close
to allow the application to disconnect with the Communication Framework.
/* implementation of MessageEndpointConnection */
public async Task Close(Exception error)
{
// await socket.Close, etc (or do nothing if already closed)
// throw MessagingException if something goes wrong (though
// since it is "close" nothing special will happen)
}
Active Peer
When the active Peer receives the corresponding disconnect notification from the Communication Framework, it must call the ReplicatorConnection.close
method.
_replicatorConnection?.Close(null);
Then, Couchbase Lite will call back your code through the MessageEndpointConnection.close
to allow the application to disconnect with the Communication Framework.
/* implementation of MessageEndpointConnection */
public async Task Close(Exception error)
{
// await socket.Close, etc (or do nothing if already closed)
// throw MessagingException if something goes wrong (though
// since it is "close" nothing special will happen)
}