User Authentication
Access {sgw} securely to sync from cloud to edge
This content explains how to implement user authentication in sync gateway
Related Security topics: TLS Certificate Authentication | Sync Function | Import filter | Read Access | Write Access
Introduction
Authentication is the process of verifying the identity of a user. Sync Gateway supports the following authentication methods:
- Anonymous Access
-
sync gateway does not allow anonymous or guest access by default, but it can be enabled by editing the configuration file or by using the Admin REST API.
- Basic Authentication
-
Provide a username and password to authenticate users.
- Auth Providers
-
sync gateway provides a turn-key solution to authenticate with Facebook or Google.
For other providers we recommend to use Custom Authentication or OpenID Connect.-
Custom Authentication Use an App Server to handle the authentication yourself and create user sessions on the sync gateway Admin REST API.
-
OpenID Connect Authentication Use OpenID Connect providers (Google+, Paypal, etc.) to authenticate users. Static providers: sync gateway currently supports authentication endpoints for Facebook, Google+ and OpenID Connect providers
-
Anonymous Access
A special user account named GUEST
applies to unauthenticated requests.
Any request to the Public REST API that does not have an Authorization
header or a session cookie is treated as coming from the GUEST
account.
This account and all anonymous access is disabled by default.
To enable the GUEST account, set its disabled
property to false.
You might also want to give it access to some channels.
If you don’t assign some channels to the GUEST account, anonymous requests won’t be able to access any documents.
The following sample command enables the GUEST account and allows it access to a channel named public.
$ curl -X PUT localhost:4985/$DB/_user/GUEST --data \
'{"disabled":false, "admin_channels":["public"]}'
Basic Authentication
Once the user has been created on sync gateway, you can provide the same username/password to the BasicAuthenticator
class of Couchbase Lite.
Under the hood, the replicator will send the credentials in the first request to retrieve a SyncGatewaySession
cookie and use it for all subsequent requests during the replication.
This is the recommended way of using basic authentication.
Auth Providers
Deprecation Notice
This feature is deprecated.It can be enabled only when running in legacy mode. |
sync gateway provides a turn-key solution to authenticate with Facebook or Google.
The app is responsible for retrieving the auth provider token and must send it to sync gateway which will return a session ID. The session ID can be used to configure the Couchbase Lite replicator or for other HTTP requests to the sync gateway REST API.
The following diagram describes the sequence of steps.
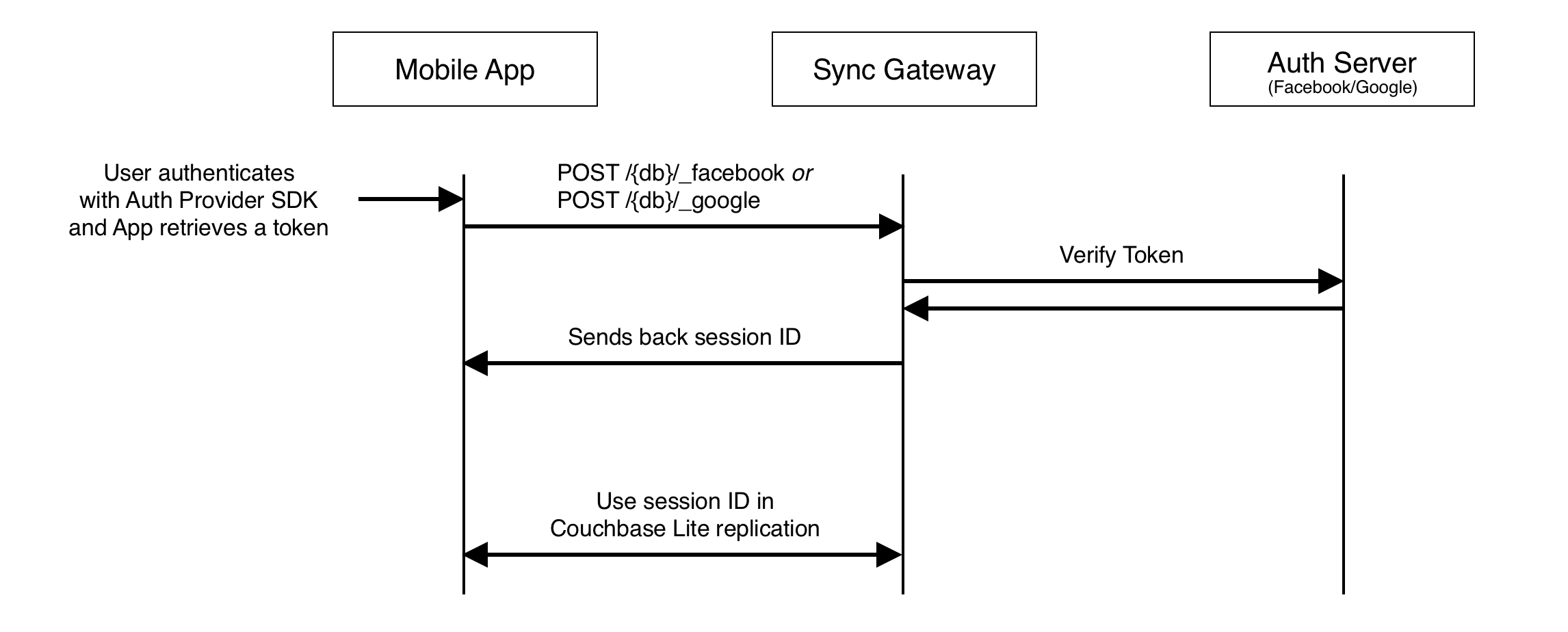
API reference:
For other providers we recommend to use Custom Authentication or OpenID Connect.
Custom Authentication
It’s possible for an application server associated with a remote Couchbase sync gateway to provide its own custom form of authentication. Generally this will involve a particular URL that the app needs to post some form of credentials to; the App Server will verify those, then tell the sync gateway to create a new session for the corresponding user, and return session credentials in its response to the client app.
The following diagram shows an example architecture to support Google SignIn in a Couchbase Mobile application, the client sends an access token to the App Server where a server side validation is done with the Google API and a corresponding sync gateway user is then created if it’s the first time the user logs in. The last request creates a session.
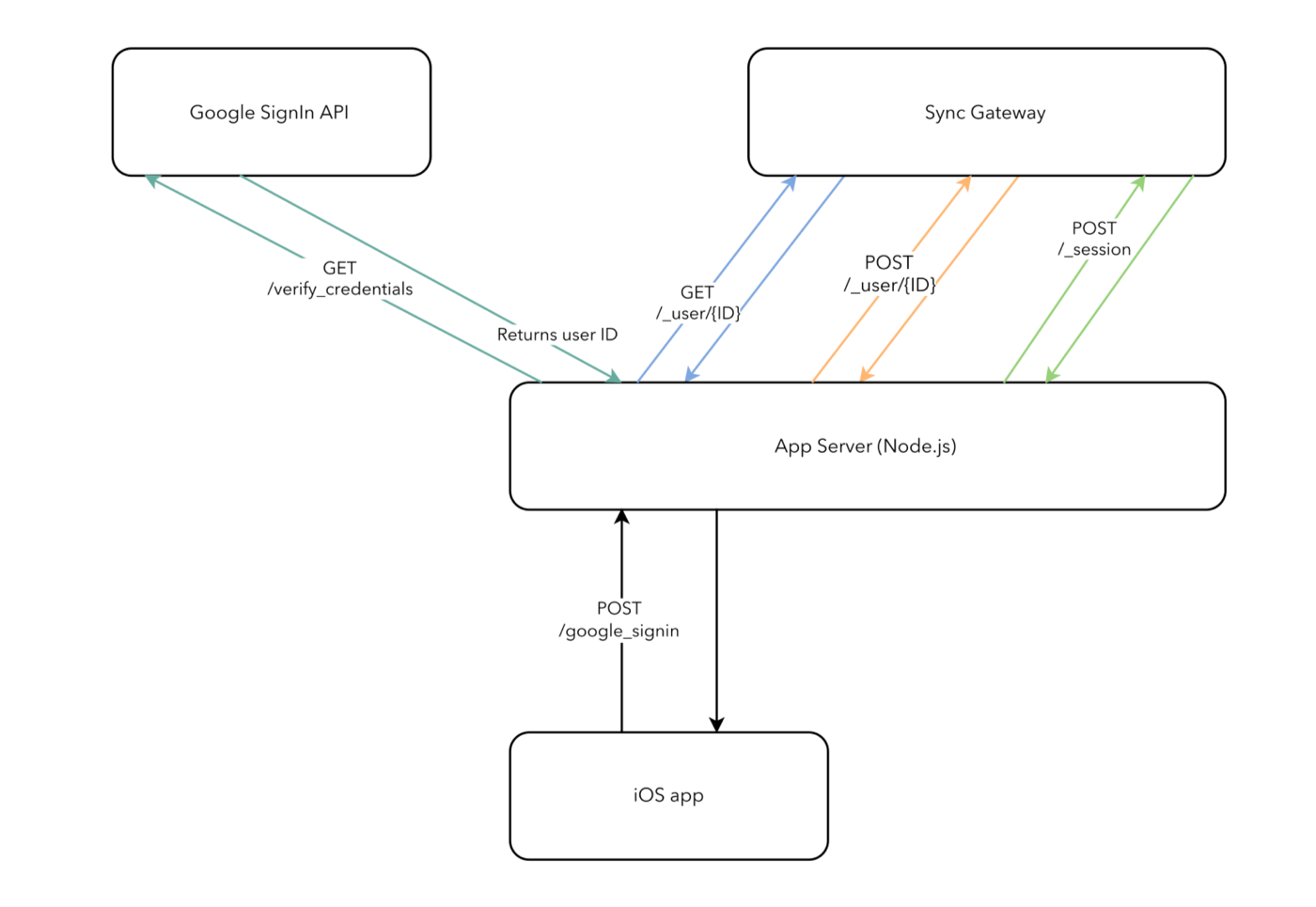
Given a user that has already been created, a new session for that user can be created on the Admin POST /\{db}/_session endpoint.
$ curl -vX POST -H 'Content-Type: application/json' \
-d '{"name": "john", "ttl": 180}' \
http://localhost:4985/sync_gateway/_session
// Response message body
{
"session_id": "904ac010862f37c8dd99015a33ab5a3565fd8447",
"expires": "2015-09-23T17:27:17.555065803+01:00",
"cookie_name": "SyncGatewaySession"
}
The HTTP response body contains the credentials of the session.
-
name corresponds to the
cookie_name
-
value corresponds to the
session_id
-
path is the hostname of the sync gateway
-
expirationDate corresponds to the cookie’s expiration time. The endpoint’s API reference contains more information about how the expiration time is automatically extended according to the user session activity.
-
secure Whether the cookie should only be sent using a secure protocol (e.g. HTTPS)
-
httpOnly Whether the cookie should only be used when transmitting HTTP, or HTTPS, requests thus restricting access from other, non-HTTP APIs
It is recommended to return the session details to the client application in the same form and to use the SessionAuthenticator
class to authenticate with that session id.
Unresolved directive in authentication-users.adoc - include::{root-partials}blocklinks-cbl.adoc[]
OpenID Connect
sync gateway supports OpenID Connect. This allows your application to use Couchbase for data synchronization and delegate the authentication to a 3rd party server (known as the Provider). There are two implementation methods available with OpenID Connect:
- Implicit Flow
-
With this method, the retrieval of the ID token takes place on the device. You can then create a user session using the POST
/\{tkn-db}/_session
endpoint on the Public REST API with the ID token. - Authorization Code Flow
-
This method relies on sync gateway to retrieve the ID token.
Implicit Flow
Implicit Flow has the key feature of allowing clients to obtain their own Open ID token and use it to authenticate against sync gateway. The implicit flow with sync gateway is as follows:
-
The client obtains a signed Open ID token directly from an OpenID Connect provider. Note that only signed tokens are supported. To verify that the Open ID token being sent is indeed signed, you can use the jwt.io Debugger. In the algorithm dropdown, make sure to select
RS256
as the signing algorithm (other options such asHS256
are not yet supported by sync gateway). -
The client includes the Open ID token as an
Authorization: Bearer <id_token>
header on requests made against the sync gateway REST API. -
sync gateway matches the token to a provider in its configuration file based on the issuer and audience in the token.
-
sync gateway validates the token, based on the provider definition.
-
Upon successful validation, sync gateway authenticates the user based on the subject and issuer in the token.
Since Open ID tokens are typically large, the usual approach is to use the Open ID token to obtain a sync gateway session id (using the POST /db/_session endpoint), and then use the returned session id for subsequent authentication requests.
Note: This example is based on a deployment using legacy configuration.
- REST API
-
curl --location --request PUT 'http://localhost:4985/ourdb/_config' \ --header 'accept: application/json' \ --header 'Content-Type: application/json' \ --data-raw '{ oidc: { providers: { google_implicit: { issuer:https://accounts.google.com, client_id:yourclientid-uso.apps.googleusercontent.com, register:true (1) }, }, } }'
- Configuration File
-
Here is a sample sync gateway config file, configured to use the Implicit Flow.
{ "databases": { "default": { "name": "dbname", "bucket": "default", "oidc": { "providers": { "google_implicit": { "issuer":"https://accounts.google.com", "client_id":"yourclientid-uso.apps.googleusercontent.com", "register":true (1) }, }, } } } }
1 | Use register to auto-register a sync gateway user on successful completion of the validation |
Client Authentication
With the implicit flow, the mechanism to refresh the token and sync gateway session must be handled in the application code.
On launch, the application should check if the token has expired.
If it has then you must request a new token (Google SignIn for iOS has a method called signInSilently
for this purpose).
By doing this, the application can then use the token to create a sync gateway session.
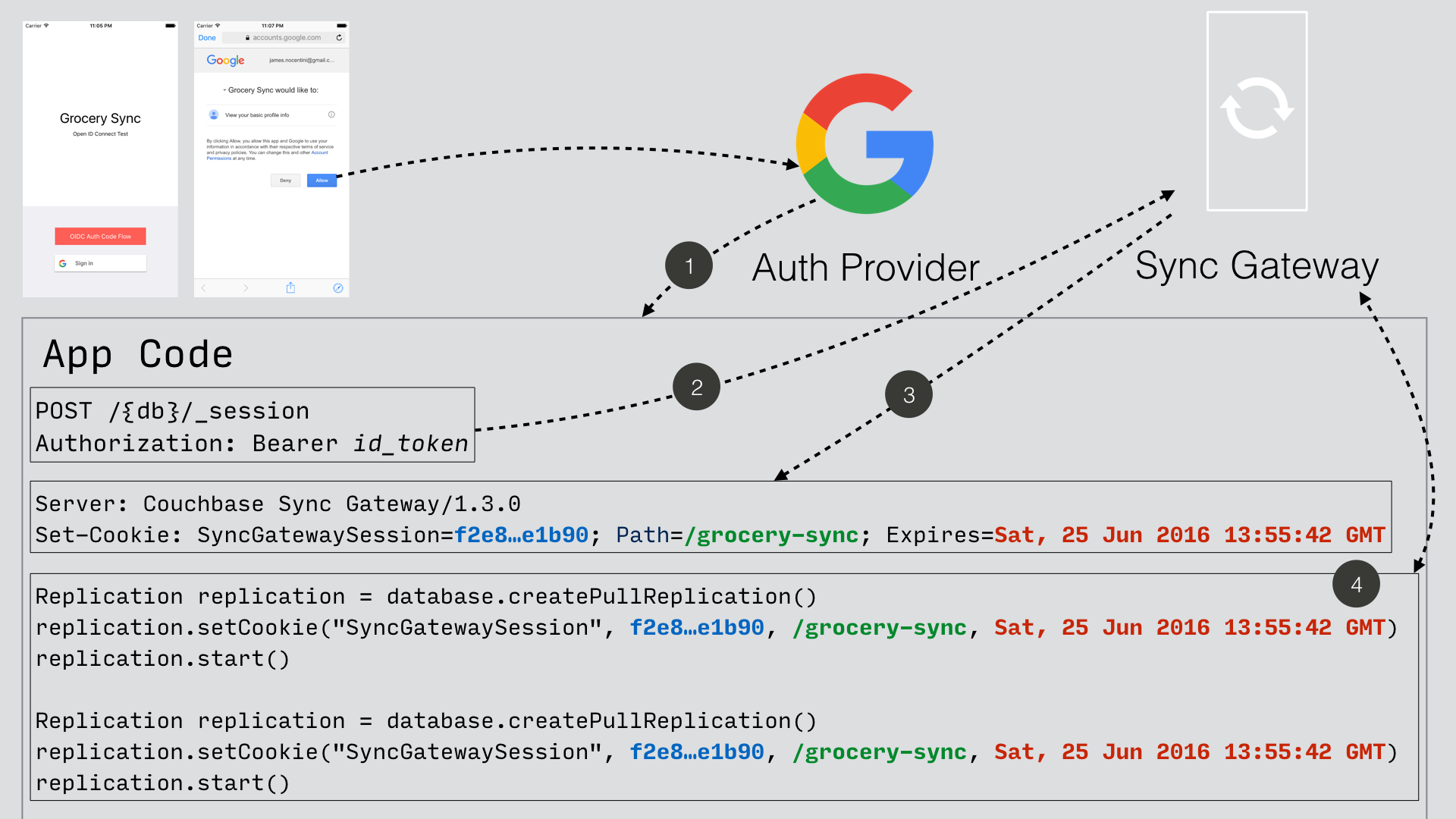
-
The Google SignIn SDK prompts the user to login and if successful it returns an ID token to the application.
-
The ID token is used to create a sync gateway session by sending a POST
/\{tkn-db}/_session
request. -
sync gateway returns a cookie session in the response header.
-
The sync gateway cookie session is used on the replicator object.
sync gateway sessions also have an expiration date.
The replication lastError
property will return a 401 Unauthorized when it’s the case and then the application must retrieve create a new sync gateway session and set the new cookie on the replicator.
A complete tutorial is available here for your reference.
You can configure your application for Google SignIn by following this guide.
Authorization Code Flow
Whilst sync gateway supports Authorization Code Flow, there is considerable work involved to implement the Authorization Code Flow on the client side.
Couchbase Lite 1.x has an API to hide this complexity called OpenIDConnectAuthenticator
but since it is not available in the 2.0 API we recommend to use the Implicit Flow.