Working with Blobs
A Blob
is an object that can appear in a document as a property value.
Just instantiate a Blob
and set it as the value of a property.
Then later get the property value, which will be a Blob
object.
The following code example adds a blob to the document under the avatar
property.
UIImage *appleImage = [UIImage imageNamed:@"avatar.jpg"];
NSData *imageData = UIImageJPEGRepresentation(appleImage, 1.0);
CBLBlob *blob = [[CBLBlob alloc] initWithContentType:@"image/jpeg" data:imageData];
[newTask setBlob:blob forKey:@"avatar"];
[database saveDocument:newTask error:&error];
CBLDocument *savedTask = [database documentWithID: @"task1"];
CBLBlob *taskBlob = [savedTask blobForKey:@"avatar"];
UIImage *taskImage = [UIImage imageWithData:taskBlob.content];
The Blob
API lets you access the contents as in-memory data (a Data
object) or as a InputStream
.
It also supports an optional type
property that by convention stores the MIME type of the contents.
In the example above, "image/jpeg" is the MIME type and "avatar" is the key which references that Blob
.
That key can be used to retrieve the Blob
object at a later time.
On Couchbase Lite, blobs can be arbitrarily large, and are only read on demand, not when you load a Document
object.
On Sync Gateway, the maximum content size is 20 MB per blob.
If a document’s blob is over 20 MB, the document will be replicated but not the blob.
When a document is synchronized, the Couchbase Lite replicator will add an _attachments
dictionary to the document’s properties if it contains a blob.
A random access name will be generated for each Blob
which is different to the "avatar" key that was used in the example above.
On the image below, the document now contains the _attachments
dictionary when viewed in the Couchbase Server Admin Console.
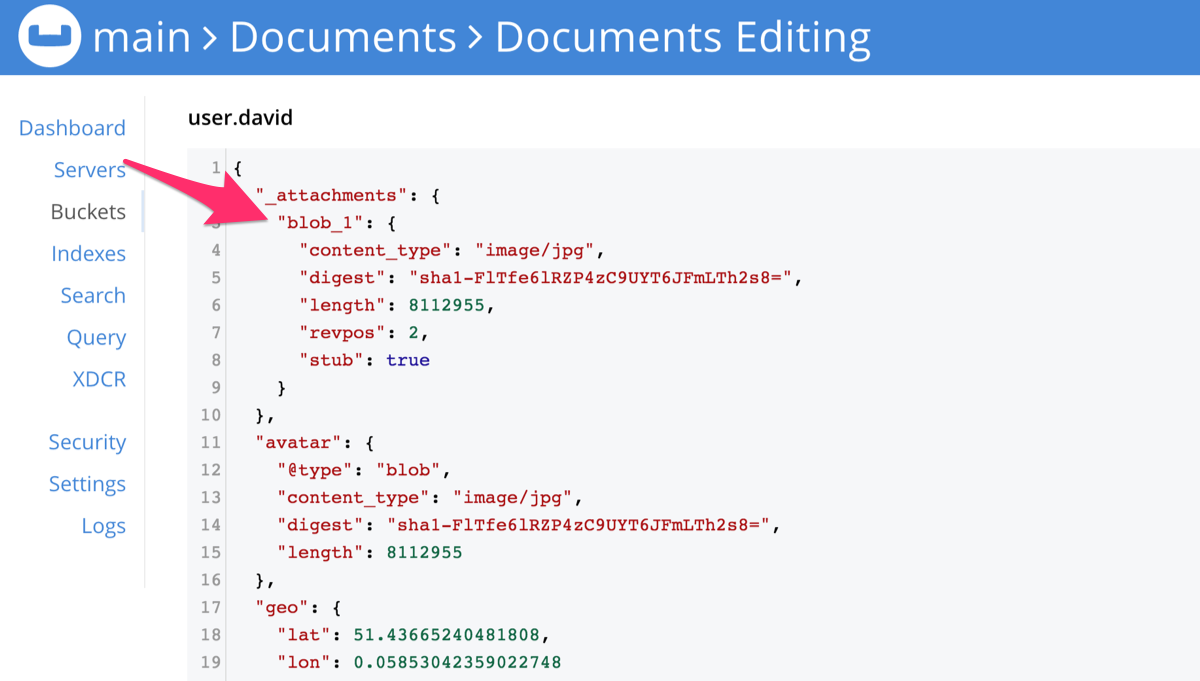
A blob also has properties such as "digest"
(a SHA-1 digest of the data), "length"
(the length in bytes), and optionally "content_type"
(the MIME type).
The data is not stored in the document, but in a separate content-addressable store, indexed by the digest.
This Blob
can be retrieved on the Sync Gateway REST API at http://localhost:4984/justdoit/user.david/blob_1.
Notice that the blob identifier in the URL path is "blob_1" (not "avatar").