Build and Run
Description — Build and run a starter app to validate your install of Couchbase Lite on Swift
You may encounter a Target Integrity Error when building for iOS Simulator(or iOS) — see Dealing with Target Integrity Error
|
Starter Code
Open ViewController.swift in Xcode and copy the following code in the viewDidLoad
method.
This snippet demonstrates how to run basic CRUD operations, a simple Query and running bi-directional replications with Sync Gateway.
// Get the database (and create it if it doesn’t exist).
let database: Database
do {
database = try Database(name: "mydb")
} catch {
fatalError("Error opening database")
}
// Create a new document (i.e. a record) in the database.
let mutableDoc = MutableDocument()
.setFloat(2.0, forKey: "version")
.setString("SDK", forKey: "type")
// Save it to the database.
do {
try database.saveDocument(mutableDoc)
} catch {
fatalError("Error saving document")
}
// Update a document.
if let mutableDoc = database.document(withID: mutableDoc.id)?.toMutable() {
mutableDoc.setString("Swift", forKey: "language")
do {
try database.saveDocument(mutableDoc)
let document = database.document(withID: mutableDoc.id)!
// Log the document ID (generated by the database)
// and properties
print("Document ID :: \(document.id)")
print("Learning \(document.string(forKey: "language")!)")
} catch {
fatalError("Error updating document")
}
}
// Create a query to fetch documents of type SDK.
let query = QueryBuilder
.select(SelectResult.all())
.from(DataSource.database(database))
.where(Expression.property("type").equalTo(Expression.string("SDK")))
// Run the query.
do {
let result = try query.execute()
print("Number of rows :: \(result.allResults().count)")
} catch {
fatalError("Error running the query")
}
// Create replicators to push and pull changes to and from the cloud.
let targetEndpoint = URLEndpoint(url: URL(string: "ws://localhost:4984/getting-started-db")!)
let replConfig = ReplicatorConfiguration(database: database, target: targetEndpoint)
replConfig.replicatorType = .pushAndPull
// Add authentication.
replConfig.authenticator = BasicAuthenticator(username: "john", password: "pass")
// Create replicator (make sure to add an instance or static variable named replicator)
self.replicator = Replicator(config: replConfig)
// Listen to replicator change events.
self.replicator.addChangeListener { (change) in
if let error = change.status.error as NSError? {
print("Error code :: \(error.code)")
}
}
// Start replication.
self.replicator.start()
Build and run. You should see the document ID and property printed to the console. The document was successfully persisted to the database.
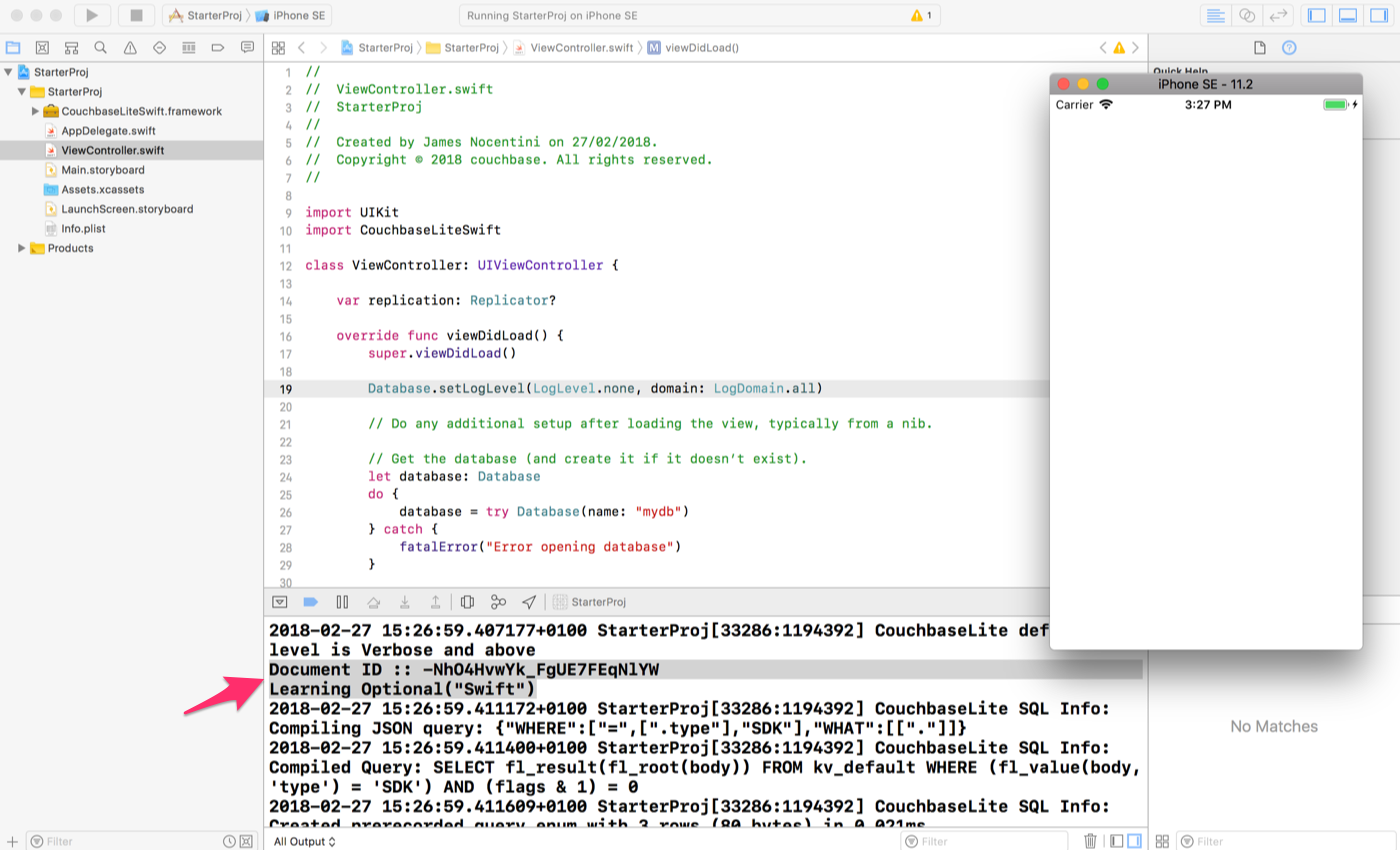
In the next step, you will setup Sync Gateway. Before synchronizing documents to Sync Gateway you will need to disable App Transport Security (ATS) on iOS. App Transport Security is enabled by default since iOS 9 and enforces applications to use HTTPS exclusively. For this getting started guide, you will disable it but we recommend to enable it in production (and enable HTTPS on Sync Gateway). In the Xcode navigator, right-click on Info.plist and open it as a source file.
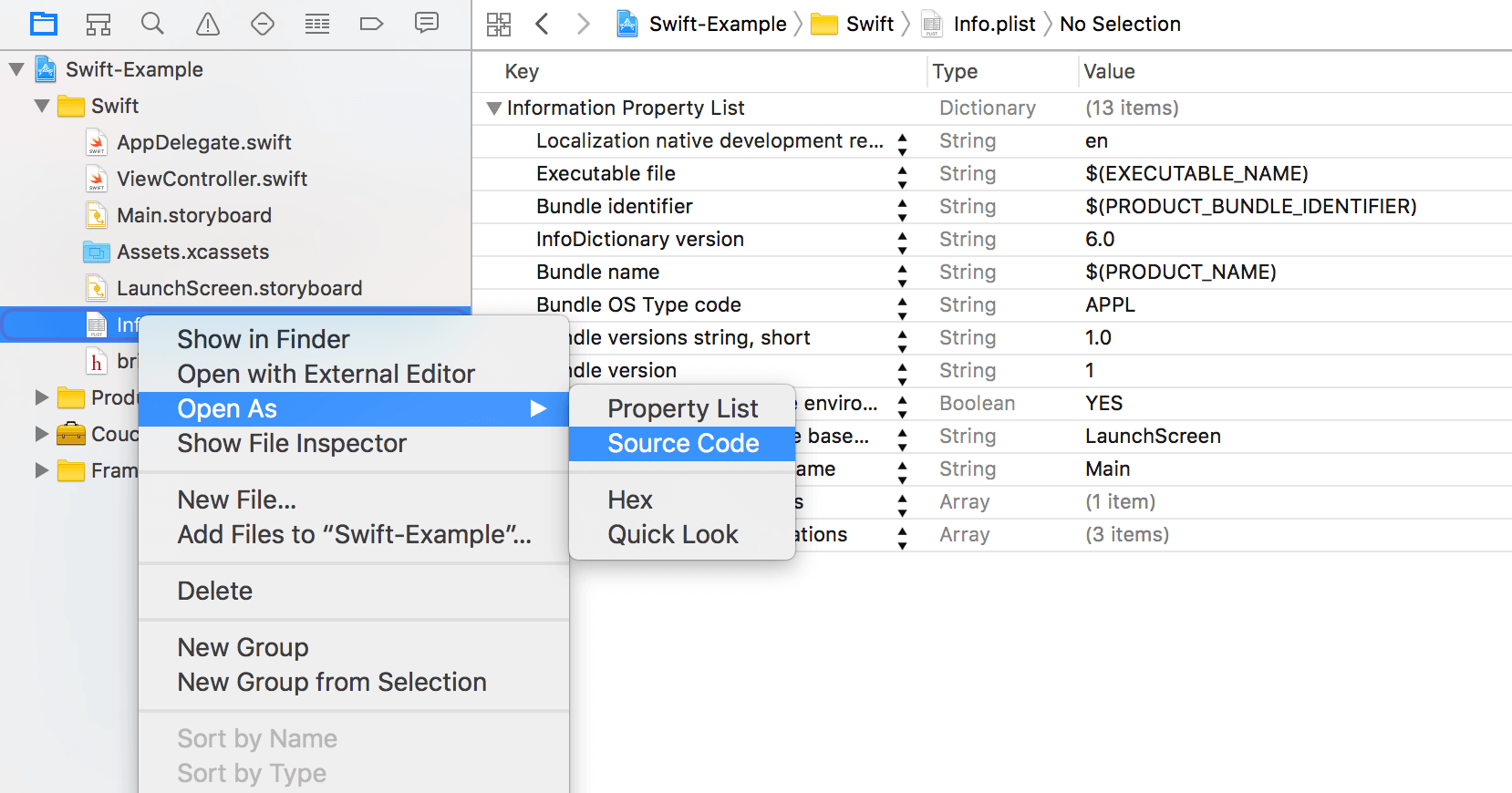
Append the following inside of the <dict>
XML tags to disable ATS.
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key><true/>
</dict>