Build and Run
Description — Build and run a starter app to validate your install of Couchbase Lite on Objective-C
Quick Steps
-
Within XCode, create a new Objective-C app project
-
Within the ViewController.m file, replace the boilerplate codein with that shown in Example 1. Note you can also get this from github.
-
Build and Run the code.
You should see the document ID and property printed to the console, indicating that the document was successfully persisted to the database as shown in Figure 1
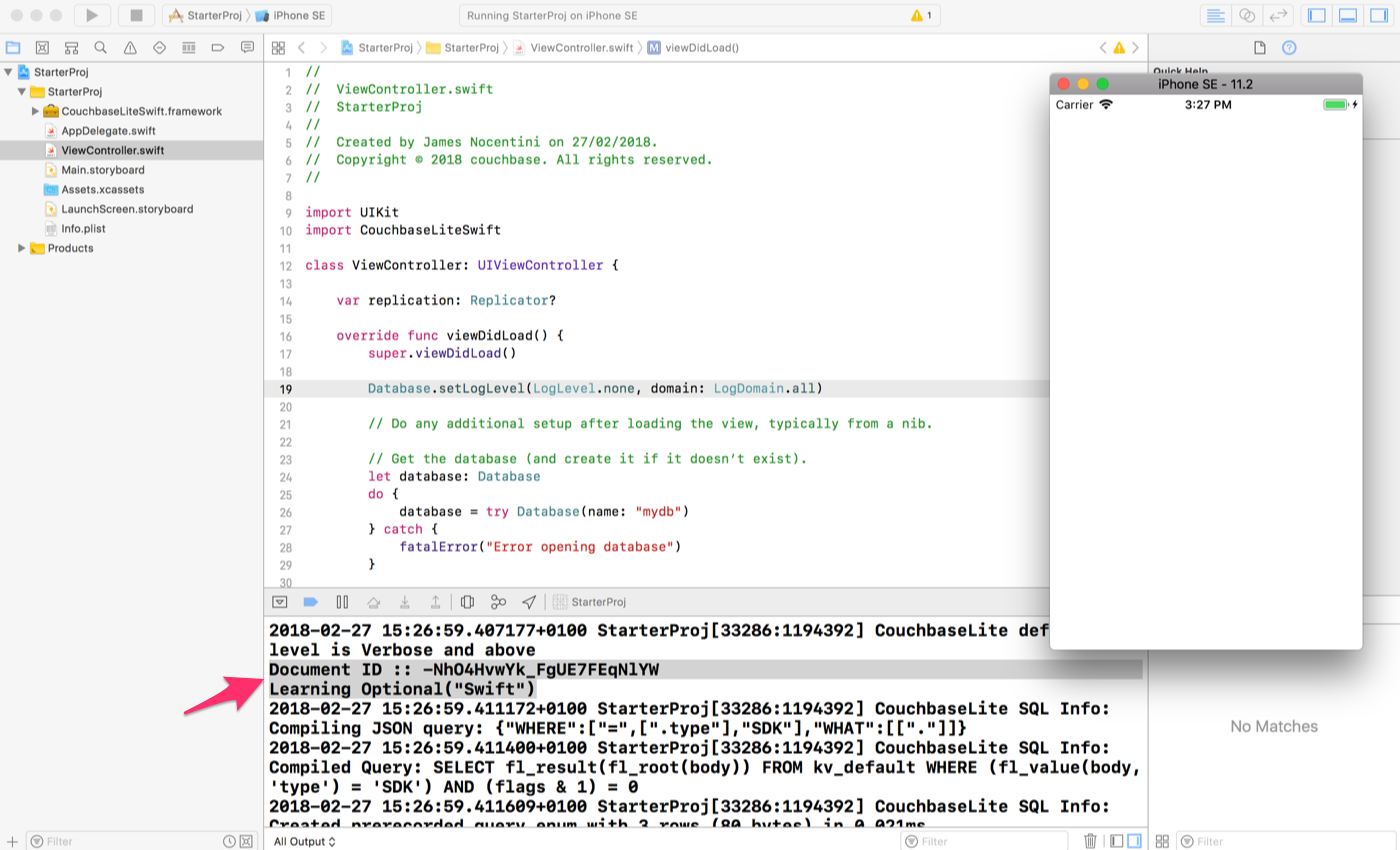
This snippet demonstrates how to run basic CRUD operations, a simple Query and running bi-directional replications with Sync Gateway.
objc//
// GettingStarted.m
//
// Copyright (c) 2024 Couchbase, Inc. All rights reserved.
//
// Licensed under the Couchbase License Agreement (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
// <a href="https://info.couchbase.com/rs/302-GJY-034/images/2017-10-30_License_Agreement.pdf" class="bare">https://info.couchbase.com/rs/302-GJY-034/images/2017-10-30_License_Agreement.pdf</a>
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
#import "GettingStarted.h"
#import <CouchbaseLite/CouchbaseLite.h>
@implementation GettingStarted
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
[self getStartedWithReplication: false];
}
- (void) getStartedWithReplication: (Boolean) replication {
NSError *error;
// Get the database (and create it if it doesn’t exist).
CBLDatabase *database = [[CBLDatabase alloc] initWithName: @"mydb" error: &error];
CBLCollection *collection = [database defaultCollection: &error];
// Create a new document (i.e. a record) in the database.
CBLMutableDocument *mutableDoc = [[CBLMutableDocument alloc] init];
[mutableDoc setFloat:2.0 forKey:@"version"];
[mutableDoc setString:@"SDK" forKey:@"type"];
// Save it to the database.
[collection saveDocument: mutableDoc error: &error];
// Update a document.
CBLMutableDocument *mutableDoc2 = [[collection documentWithID: mutableDoc.id error: &error] toMutable];
[mutableDoc2 setString: @"Objective-C" forKey: @"language"];
[collection saveDocument: mutableDoc2 error: &error];
CBLDocument *document = [collection documentWithID: mutableDoc2.id error: &error];
// Log the document ID (generated by the database)
// and properties
NSLog(@"Document ID :: %@", document.id);
NSLog(@"Learning %@", [document stringForKey:@"language"]);
// Create a query to fetch documents of type SDK.
CBLQueryExpression *type = [[CBLQueryExpression property:@"type"] equalTo:[CBLQueryExpression string:@"SDK"]];
CBLQuery *query = [CBLQueryBuilder select:@[[CBLQuerySelectResult all]]
from:[CBLQueryDataSource collection: collection]
where:type];
// Run the query
CBLQueryResultSet *result = [query execute:&error];
NSLog(@"Number of rows :: %lu", (unsigned long)[[result allResults] count]);
if (replication) {
// Create replicators to push and pull changes to and from the cloud.
NSURL *url = [[NSURL alloc] initWithString:@"ws://localhost:4984/getting-started-db"];
CBLURLEndpoint *targetEndpoint = [[CBLURLEndpoint alloc] initWithURL:url];
CBLReplicatorConfiguration *replConfig = [[CBLReplicatorConfiguration alloc] initWithTarget:targetEndpoint];
replConfig.replicatorType = kCBLReplicatorTypePushAndPull;
// Add authentication.
replConfig.authenticator = [[CBLBasicAuthenticator alloc] initWithUsername:@"john" password:@"pass"];
// Add collection
[replConfig addCollection: collection config: nil];
// Create replicator
CBLReplicator *_replicator = [[CBLReplicator alloc] initWithConfig: replConfig];
// Listen to replicator change events.
[_replicator addChangeListener:^(CBLReplicatorChange *change) {
if (change.status.error) {
NSLog(@"Error code: %ld", change.status.error.code);
}
}];
// Start replication
[_replicator start];
} else {
NSLog(@"Not running replication");
}
}
@end
Before synchronizing documents to Sync Gateway you will need to disable App Transport Security. In the Xcode navigator, right-click on Info.plist and open it as a source file.
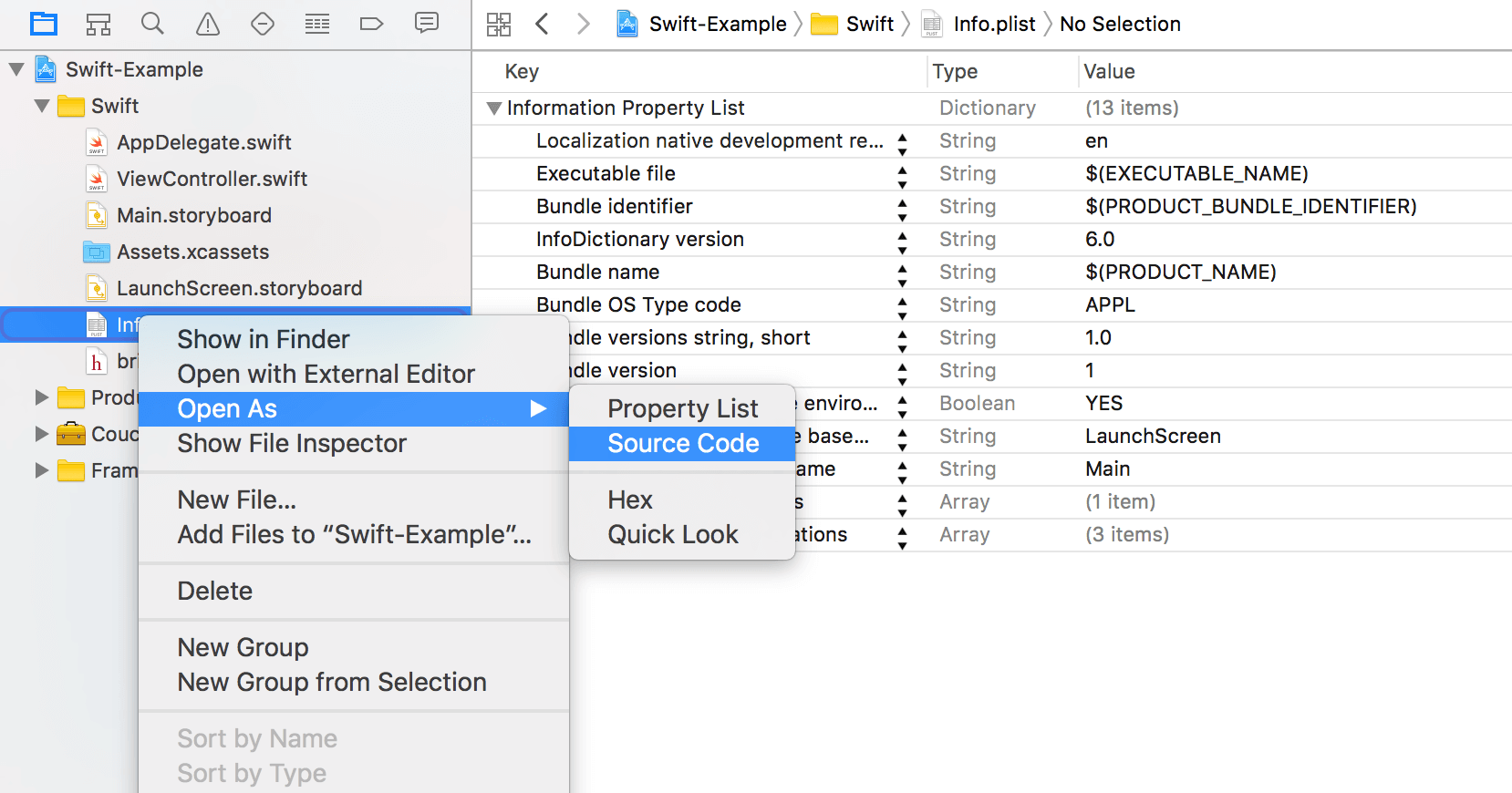
Append the following inside of the <dict>
XML tags to disable Application Transport Security (ATS). The ATS must be disabled because the sample code snippet uses the WebSocket protocol (WS://) over the unencrypted HTTP protocol — and would conflict with ATS’s security requirements.
xml<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key><true/>
</dict>
See: Install Sync Gateway