Create Couchbase Transactions with SQL++
- how-to
How to create Couchbase transactions using SQL++.
Introduction
Couchbase transactions enable you to carry out ACID (atomic, consistent, isolated, and durable) actions on the database. This how-to guide covers SQL++ support for Couchbase transactions. Some SDKs also support Couchbase transactions. Refer to Related Links for further details.
Only DML (data modification language) statements are permitted within a transaction: INSERT, UPSERT, DELETE, UPDATE, MERGE, SELECT, EXECUTE FUNCTION, PREPARE, or EXECUTE.
If you want to try out the examples in this section, follow the instructions given in Do a Quick Install to install Couchbase Server, configure a cluster, and load a sample dataset. Read the following for further information about the tools available for editing and executing queries:
Please note that the examples in this guide will alter the data in your sample database. To restore your sample data, remove and reinstall the travel sample data. Refer to Sample Buckets for details. |
Transaction Parameters
You can specify various settings and parameters to control how transactions work. You can access transaction settings and parameters through any of the usual Query tools, such as the Query Workbench or the cbq shell.
-
Query Workbench
-
CBQ Shell
To specify parameters for a Couchbase transaction, use the Query Run-Time Preferences window.
-
To display the Run-Time Preferences window, click the cog icon .
-
To specify the transaction scan consistency, open the Scan Consistency drop-down list and select an option.
-
To specify the transaction timeout, in the Transaction Timeout box, enter a value in seconds.
-
To specify any other transaction parameters, click the + button in the Named Parameters section. When the new named parameter appears, enter the name in the name box and a value in the value box.
-
To save the preferences and return to the Query Workbench, click Save Preferences.
The following example shows transaction parameters for the examples on this page.
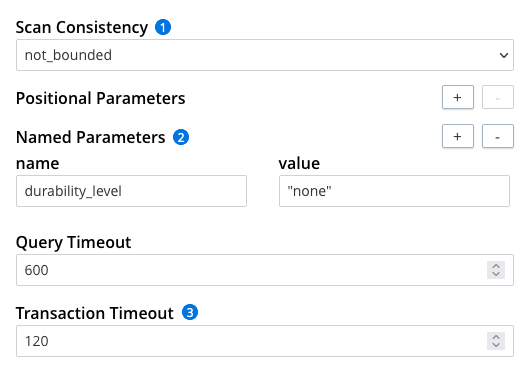
-
The transaction scan consistency is set to
not_bounded
. -
The durability level of all the mutations within the transaction is set to
"none"
. -
The transaction timeout is set to
120
.
To specify parameters for a Couchbase transaction, use the \SET
command.
The following example shows transaction parameters for the examples on this page.
\SET -txtimeout "2m"; (1)
\SET -scan_consistency "not_bounded"; (2)
\SET -durability_level "none"; (3)
1 | The transaction timeout. |
2 | The transaction scan consistency. |
3 | Durability level of all the mutations within the transaction. |
Click the View button to see this code in context.
For further details, refer to Transaction Settings and Parameters.
Single Statement Transactions
You can create a Couchbase transaction containing a single DML statement.
-
Query Workbench
-
CBQ Shell
To execute a single statement as a transaction, simply enter the statement in the Query Editor and click Run as TX.
Set the query context to the inventory
scope in the travel sample dataset.
For more information, see Setting the Query Context.
UPDATE hotel
SET price = "from £89"
WHERE name = "Glasgow Grand Central";
To execute a single statement as a transaction, set the tximplicit
parameter to true
.
Set the query context to the inventory
scope in the travel sample dataset.
For more information, see Setting the Query Context.
\SET -tximplicit true;
UPDATE hotel
SET price = "from £89"
WHERE name = "Glasgow Grand Central";
For further details, refer to Query Tools.
Multiple Statement Transactions
A Couchbase transaction may contain multiple DML statements. In this case, you must use SQL++ transaction statements to support the transaction:
-
BEGIN TRANSACTION to start the transaction.
-
SET TRANSACTION to specify transaction settings.
-
SAVEPOINT to set a transaction savepoint.
-
ROLLBACK TRANSACTION to roll back a transaction.
-
COMMIT TRANSACTION to commit a transaction.
-
Query Workbench
-
CBQ Shell
To execute a transaction containing multiple statements:
-
Compose the sequence of statements in the Query Editor. Each statement must be terminated with a semicolon.
-
After each statement, press Shift+Enter to start a new line without executing the query.
-
When you have entered the entire transaction, click Execute to execute the transaction.
For a worked example showing a complete transaction using SQL++, see Transaction Worked Example. Individual SQL++ transaction statements are described in the sections below.
To execute a transaction containing multiple statements, create the transaction one statement at a time.
Once you have started a transaction, all statements within the cbq shell session are assumed to be part of the same transaction until you rollback or commit the transaction.
You must be using cbq shell version 2.0 or above to use the automatic transaction ID functionality. |
For a worked example showing a complete transaction using SQL++, see Transaction Worked Example. Individual SQL++ transaction statements are described in the sections below.
Begin a Transaction
To start a transaction, use the BEGIN TRANSACTION
statement.
The following statement begins a transaction.
BEGIN WORK;
Click the View button to see this code in context.
{
"txid": "d81d9b4a-b758-4f98-b007-87ba262d3a51" (1)
}
1 | Beginning a transaction returns the transaction ID. |
For further details, refer to BEGIN TRANSACTION.
Specify Transaction Settings
To specify transaction settings, use the SET TRANSACTION
statement.
Currently, the only available transaction setting is "isolation level read committed".
This setting is enabled by default.
The SET TRANSACTION statement is therefore optional and may be omitted.
|
The following statement specifies transaction settings.
SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
Click the View button to see this code in context.
For further details, refer to SET TRANSACTION.
Set a Savepoint
To set a savepoint within a transaction, use the SAVEPOINT
statement and specify a name for the savepoint.
The following statement sets a savepoint.
SAVEPOINT s1;
Click the View button to see this code in context.
For further details, refer to SAVEPOINT.
Roll Back a Transaction
To roll back a transaction, use the ROLLBACK TRANSACTION
statement.
By default, this statement rolls back the entire transaction.
If you want to roll back to a savepoint, use the TO SAVEPOINT
keywords and specify the savepoint name.
The following statement rolls back a transaction to a specified savepoint.
ROLLBACK TRAN TO SAVEPOINT s2;
Click the View button to see this code in context.
For further details, refer to ROLLBACK TRANSACTION.
Commit a Transaction
To commit a transaction, use the COMMIT TRANSACTION
statement.
The following statement commits a transaction.
COMMIT WORK;
Click the View button to see this code in context.
For further details, refer to COMMIT TRANSACTION.