Connect to Couchbase Server
- how-to
How to connect to a Couchbase Cluster.
Introduction
Connecting to Couchbase can be done in several ways. This guide will take you through some of the most common methods used to access a Couchbase cluster with an SDK client or CLI tool.
A Couchbase Cluster is a combination of multiple server nodes, which can be accessed by users or applications with a username and password. Each server node can also be its own cluster or join an existing multi-node setup.
Couchbase uses Role Based Access Control (RBAC) to control access to its various services. A user or application can connect to a cluster and use these services, assuming that valid credentials with relevant access roles are provided.
Before You Begin
If you want to try out the examples in this section, follow the instructions given in Do a Quick Install to install Couchbase Server, configure a cluster, and load a sample dataset.
Couchbase Clients
Clients access data by connecting to a Couchbase cluster over the network. The most common type of client is a Couchbase SDK, which is a full programmatic API that enables applications to take the best advantage of Couchbase. This developer guide focuses on the most commonly-used SDKs, but full explanations and reference documentation for all SDKs is available.
The command line clients also provide a quick and streamlined interface for simple access and are suitable if you just want to access an item without writing any code.
With some editions, the command line clients are provided as part of the installation of Couchbase Server. Assuming a default installation, you can find them in the following location, depending on your operating system:
If the command line client is not provided with your installation of Couchbase Server, you must install the C SDK in order to use the command line clients. |
Read the following for further information about the clients available:
Connecting via the Web Console
To access a cluster via the Couchbase Server Web Console over an unencrypted connection, navigate to the Web Console address with your browser (by default, http://localhost:8091
) and enter your credentials.
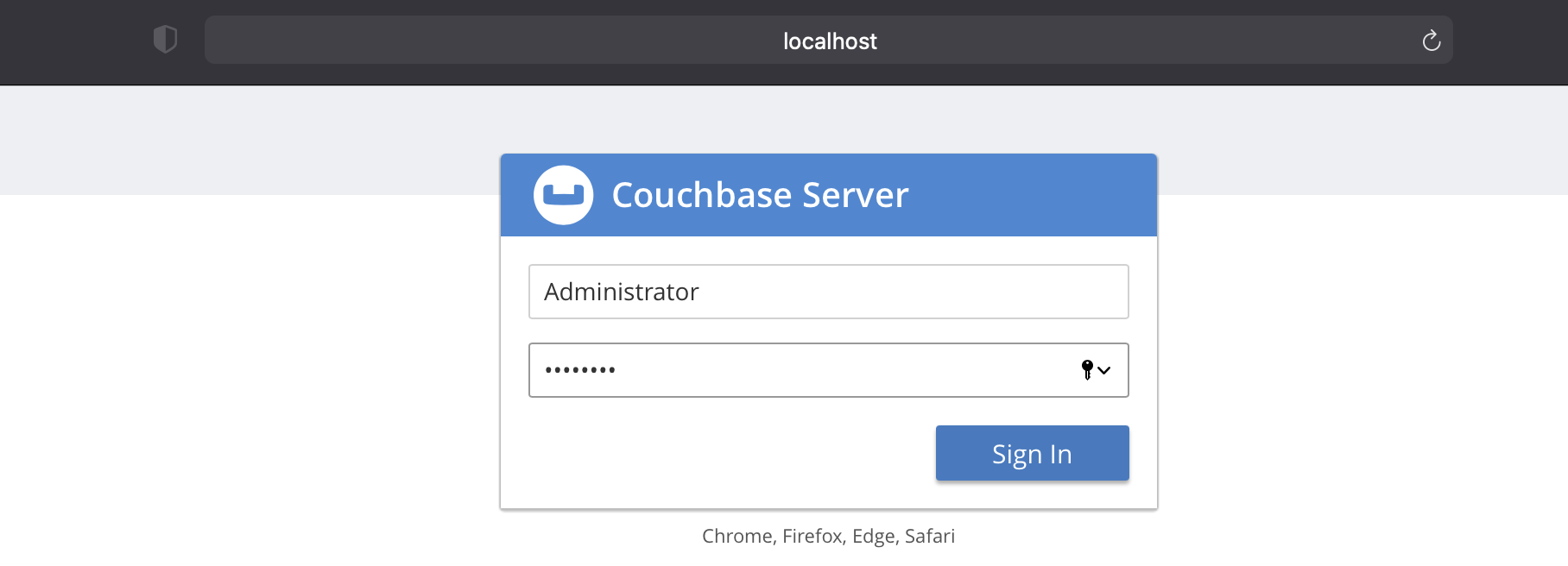
See Authenticating with the Console for more information.
To access a cluster via the Couchbase Server Web Console over an encrypted connection, navigate to the secure Web Console address with your browser (by default, https://localhost:18091
) and enter your credentials.
See Manage Console Access for more information.
Connecting via Client
Couchbase Server can be configured to run with unencrypted or encrypted network access. When running Couchbase in a production environment, the latter is always recommended.
Basic Authentication
To connect to a standalone or Docker installation with unencrypted network access, set up a user with appropriate access levels and a secure password.
-
cbc
-
.NET
-
Java
-
Node.js
-
Python
Most cbc
sub-commands will require some form of authentication to access a cluster or perform operations on data within a bucket.
-
To connect to Couchbase Server using
cbc
, pass-u
for the username,-P
for the password and-U
for the connection URL immediately after a sub-command. -
Provide the bucket name required in the connection URL (i.e., couchbase://localhost/<bucket-name>).
The example below connects to the travel-sample
bucket with Admin level credentials and performs a ping
to check what services are running in a single-node cluster environment.
cbc ping -u Administrator -P password -U couchbase://localhost/travel-sample \
--count=1 \
--table
-------------------------------------------------------------------------------
| type | id | status | latency, us | remote | local |
-------------------------------------------------------------------------------
| cbas | 0xec22b0 | ok | 3003 | localhost:8095 | 127.0.0.1:38612 |
| fts | 0xec0dc0 | ok | 3842 | localhost:8094 | 127.0.0.1:35636 |
| kv | 0xeaa220 | ok | 4446 | localhost:11210 | 127.0.0.1:49426 |
| n1ql | 0xead260 | ok | 4249 | localhost:8093 | 127.0.0.1:56740 |
| views | 0xec0430 | ok | 4045 | localhost:8092 | 127.0.0.1:60088 |
-------------------------------------------------------------------------------
If the user credentials are invalid, cbc will return a LCB_ERR_AUTHENTICATION_FAILURE error.
|
For further details, refer to cbc(1).
Call the Cluster.ConnectAsync()
method with a connection URL, username and password.
The example below connects to a single-node cluster environment with basic auth credentials.
var cluster = await Cluster.ConnectAsync("couchbase://your-ip", "Administrator", "password");
var bucket = await cluster.BucketAsync("travel-sample");
var collection = bucket.DefaultCollection();
// You can access multiple buckets using the same Cluster object.
var anotherBucket = await cluster.BucketAsync("travel-sample");
// You can access collections other than the default
// if your version of Couchbase Server supports this feature.
var inventory = bucket.Scope("inventory");
var airline = inventory.Collection("airline");
// For a graceful shutdown, disconnect from the cluster when the program ends.
await cluster.DisposeAsync();
Click the View button to see this code in context.
For further details, refer to Cluster.
Call the Cluster.connect()
method with a connection URL, username and password.
The example below connects to a single-node cluster environment with basic auth credentials.
Cluster cluster = Cluster.connect("127.0.0.1", "username", "password");
Bucket bucket = cluster.bucket("travel-sample");
Collection collection = bucket.defaultCollection();
// You can access multiple buckets using the same Cluster object.
Bucket anotherBucket = cluster.bucket("beer-sample");
// You can access collections other than the default
// if your version of Couchbase Server supports this feature.
Scope customerA = bucket.scope("customer-a");
Collection widgets = customerA.collection("widgets");
// For a graceful shutdown, disconnect from the cluster when the program ends.
cluster.disconnect();
If the user credentials provided are invalid, the SDK will return a AuthenticationFailureException error.
|
Click the View button to see this code in context.
For further details, refer to Cluster.
Call the connect()
function with a connection URL, and a ConnectOptions
object containing the username and password.
The example below connects to a single-node cluster environment with basic auth credentials.
var cluster = await couchbase.connect('couchbase://localhost', {
username: 'Administrator',
password: 'password',
})
If the user credentials provided are invalid, the SDK will return a AuthenticationFailureError error.
|
Click the View button to see this code in context.
For further details, refer to Cluster.
-
Call the
Cluster.connect()
function with a connection URL, and aClusterOptions
object containing aPasswordAutheticator
. -
Provide a username and password to the
PasswordAutheticator
.
The example below connects to a single-node cluster environment with basic auth credentials.
cluster = Cluster.connect("couchbase://your-ip", ClusterOptions(PasswordAuthenticator("Administrator", "password")))
bucket = cluster.bucket("travel-sample")
collection = bucket.default_collection()
# You can access multiple buckets using the same Cluster object.
another_bucket = cluster.bucket("beer-sample")
# You can access collections other than the default
# if your version of Couchbase Server supports this feature.
customer_a = bucket.scope("customer-a")
widgets = customer_a.collection("widgets")
If the user credentials provided are invalid, the SDK will return a AuthenticationException error.
|
Click the View button to see this code in context.
For further details, refer to Cluster.
TLS Authentication
To connect to a cluster configured with TLS (Transport Layer Security), for encrypted network access, you must supply an X.509 certificate. Before using X.509 certificate based authentication, you should run through the following:
-
cbc
-
.NET
-
Java
-
Node.js
-
Python
-
To securely connect to Couchbase Server using
cbc
, pass-U
for the connection URL immediately after a sub-command. -
Provide the bucket name required in the connection URL (i.e., couchbases://127.0.0.1/<bucket-name>). Note that "couchbases://" implies an encrypted connection scheme is used.
-
Pass a
certpath
query parameter to the connection URL, after the bucket name.
The example below connects to the travel-sample
bucket with a client certificate, and performs a ping
to check what services are running in a single-node secured cluster environment.
cbc ping -v -U "couchbases://127.0.0.1/travel-sample?certpath=ca.pem" \
--count=1 \
--table
For further details, refer to cbc(1).
-
Call the
WithX509CertificateFactory()
method on aClusterOptions()
object. -
Provide the certificate store information to the
WithX509CertificateFactory()
method. -
Call the
ConnectAsync()
method and pass it the cluster options object.
The example below connects to a single-node cluster over a secure connection with a client certificate.
It is assumed that a valid client certificate and certificate store have been set up.
var options = new ClusterOptions();
options.WithX509CertificateFactory(CertificateFactory.GetCertificatesFromStore(
new CertificateStoreSearchCriteria
{
FindValue = "value",
X509FindType = X509FindType.FindBySubjectName,
StoreLocation = StoreLocation.CurrentUser,
StoreName = StoreName.CertificateAuthority
}));
options.WithConnectionString("couchbase://your-ip");
options.WithCredentials("Administrator", "password");
var cluster = await Cluster.ConnectAsync(options);
Click the View button to see this code in context.
For further details, refer to Cluster.
-
Load a Java keystore file containing your client certificate and pass it to the
CertificateAuthenticator.fromKeyStore()
method along with the required password. -
Call the
Cluster.connect()
method and pass the connection string along with cluster options containing theCertificateAuthenticator
object previously created.
The example below connects to a single-node cluster over a secure connection with a client certificate.
It is assumed that a valid client certificate and a Java keystore have been set up.
// Replace the following line with code that gets your actual key store.
// The key store contains the client's certificate and private key.
KeyStore keyStore = loadKeyStore();
Authenticator authenticator = CertificateAuthenticator.fromKeyStore(
keyStore,
"keyStorePassword"
);
Cluster cluster = Cluster.connect(
"couchbases://127.0.0.1",
clusterOptions(authenticator)
.environment(env -> env
.securityConfig(security -> security
// Tell the client to trust the cluster's root certificate.
// If your cluster's root certificate is from a well-known
// Certificate Authority (CA), you can skip this.
.trustCertificate(Paths.get("/path/to/ca-cert.pem"))
)
)
);
Click the View button to see this code in context.
For further details, refer to Cluster.
Call the connect()
function with a connection URL, and a ConnectOptions
object containing a certificate trustStorePath
and user credentials.
The example below connects to a single-node cluster over a secure connection with a client certificate.
It is assumed that a valid client certificate has been set up.
cluster = await couchbase.connect('couchbases://localhost', {
trustStorePath: '/path/to/ca/certificates.pem',
username: 'Administrator',
password: 'password',
})
Click the View button to see this code in context.
For further details, refer to Cluster.
-
Call the
Cluster.connect()
function with a connection URL, and aClusterOptions
object containing aPasswordAutheticator
. -
Provide a username and password to the
PasswordAutheticator
. -
Supply a certificate path to the
PasswordAutheticator
.
The example below connects to a single-node cluster over a secure connection with a client certificate.
It is assumed that a valid client certificate has been set up.
cluster = Cluster("couchbases://your-ip",ClusterOptions(PasswordAuthenticator("Administrator","password",cert_path="/path/to/cluster.crt")))
Click the View button to see this code in context.
For further details, refer to Cluser.